- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free

View Transitions API
Limited availability.
This feature is not Baseline because it does not work in some of the most widely-used browsers.
- See full compatibility
- Report feedback
Experimental: This is an experimental technology Check the Browser compatibility table carefully before using this in production.
The View Transitions API provides a mechanism for easily creating animated transitions between different DOM states while also updating the DOM contents in a single step.
Concepts and usage
View transitions are a popular design choice for reducing users' cognitive load, helping them stay in context, and reducing perceived loading latency as they move between states or views of an application.
However, creating view transitions on the web has historically been difficult. Transitions between states in single-page apps (SPAs) tend to involve writing significant CSS and JavaScript to:
- Handle the loading and positioning of the old and new content.
- Animate the old and new states to create the transition.
- Stop accidental user interactions with the old content from causing problems.
- Remove the old content once the transition is complete.
Accessibility issues like loss of reading position, focus confusion, and strange live region announcement behavior can also result from having the new and old content both present in the DOM at once. In addition, cross-document view transitions (i.e. across different pages in regular, non-SPA websites) are impossible.
The View Transitions API provides a much easier way of handling the required DOM changes and transition animations.
Note: The View Transitions API doesn't currently enable cross-document view transitions, but this is planned for a future level of the spec and is actively being worked on.
Creating a basic view transition
As an example, an SPA may include functionality to fetch new content and update the DOM in response to an event of some kind, such as a navigation link being clicked or an update being pushed from the server. In our Basic View Transitions demo we've simplified this to a displayNewImage() function that shows a new full-size image based on the thumbnail that was clicked. We've encapsulated this inside an updateView() function that only calls the View Transition API if the browser supports it:
This code is enough to handle the transition between displayed images. Supporting browsers will show the change from old to new images and captions as a smooth cross-fade (the default view transition). It will still work in non-supporting browsers but without the nice animation.
It is also worth mentioning that startViewTransition() returns a ViewTransition instance, which includes several promises, allowing you to run code in response to different parts of the view transition process being reached.
The view transition process
Let's walk through how this works:
- When document.startViewTransition() is called, the API takes a screenshot of the current page.
- Next, the callback passed to startViewTransition() is invoked, in this case displayNewImage , which causes the DOM to change. When the callback has run successfully, the ViewTransition.updateCallbackDone promise fulfills, allowing you to respond to the DOM updating.
- The API captures the new state of the page as a live representation.
- ::view-transition is the root of view transitions overlay, which contains all view transitions and sits over the top of all other page content.
- ::view-transition-old is the screenshot of the old page view, and ::view-transition-new is the live representation of the new page view. Both of these render as replaced content, in the same manner as an <img> or <video> , meaning that they can be styled with handy properties like object-fit and object-position .
- The old page view animates from opacity 1 to 0, while the new view animates from opacity 0 to 1, which is what creates the default cross-fade.
- When the transition animation has reached its end state, the ViewTransition.finished promise fulfills, allowing you to respond.
Note: If the document's page visibility state is hidden (for example if the document is obscured by a window, the browser is minimized, or another browser tab is active) during a document.startViewTransition() call, the view transition is skipped entirely.
Different transitions for different elements
At the moment, all of the different elements that change when the DOM updates are transitioned using the same animation. If you want different elements to animate differently from the default "root" animation, you can separate them out using the view-transition-name property. For example:
We've given the <figcaption> element a view-transition-name of figure-caption to separate it from the rest of the page in terms of view transitions.
With this CSS applied, the pseudo-element tree will now look like this:
The existence of the second set of pseudo-elements allows separate view transition styling to be applied just to the <figcaption> . The different old and new page view captures are handled completely separate from one another.
The value of view-transition-name can be anything you want except for none — the none value specifically means that the element will not participate in a view transition.
Note: view-transition-name must be unique. If two rendered elements have the same view-transition-name at the same time, ViewTransition.ready will reject and the transition will be skipped.
Customizing your animations
The View Transitions pseudo-elements have default CSS Animations applied (which are detailed in their reference pages ).
Notably, transitions for height , width , position , and transform do not use the smooth cross-fade animation. Instead, height and width transitions apply a smooth scaling animation. Meanwhile, position and transform transitions apply smooth movement animations to the element.
You can modify the default animation in any way you want using regular CSS.
For example, to change the speed of it:
Let's look at something more interesting — a custom animation for the <figcaption> :
Here we've created a custom CSS animation and applied it to the ::view-transition-old(figure-caption) and ::view-transition-new(figure-caption) pseudo-elements. We've also added a number of other styles to both to keep them in the same place and stop the default styling from interfering with our custom animations.
Note that we also discovered another transition option that is simpler and produced a nicer result than the above. Our final <figcaption> view transition ended up looking like this:
This works because, by default, ::view-transition-group transitions width and height between the old and new views. We just needed to set a fixed height on both states to make it work.
Note: Smooth and simple transitions with the View Transitions API contains several other customization examples.
Controlling animations with JavaScript
The document.startViewTransition() method returns a ViewTransition object instance, which contains several promise members allowing you to run JavaScript in response to different states of the transition being reached. For example, ViewTransition.ready fulfills once the pseudo-element tree is created and the animation is about to start, whereas ViewTransition.finished fulfills once the animation is finished, and the new page view is visible and interactive to the user.
For example, the following JavaScript could be used to create a circular reveal view transition emanating from the position of the user's cursor on click, with animation provided by the Web Animations API .
This animation also requires the following CSS, to turn off the default CSS animation and stop the old and new view states from blending in any way (the new state "wipes" right over the top of the old state, rather than transitioning in):
Represents a view transition, and provides functionality to react to the transition reaching different states (e.g. ready to run the animation, or animation finished) or skip the transition altogether.
Extensions to other interfaces
Starts a new view transition and returns a ViewTransition object to represent it.
CSS additions
Provides the selected element with a separate identifying name and causes it to participate in a separate view transition from the root view transition — or no view transition if the none value is specified.
Pseudo-elements
The root of the view transitions overlay, which contains all view transitions and sits over the top of all other page content.
The root of a single view transition.
The container for a view transition's old and new views — before and after the transition.
A static screenshot of the old view, before the transition.
A live representation of the new view, after the transition.
- Basic View Transitions demo : A basic image gallery demo with separate transitions between old and new images, and old and new captions.
- HTTP 203 playlist : A more sophisticated video player demo app that features a number of different view transitions, many of which are explained in Smooth and simple transitions with the View Transitions API .
Specifications
Browser compatibility, api.document.startviewtransition.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
api.ViewTransition
- Smooth and simple transitions with the View Transitions API
- View Transitions API: Creating Smooth Page Transitions
- Español – América Latina
- Português – Brasil
- Tiếng Việt
- Web Platform
Smooth and simple transitions with the View Transitions API
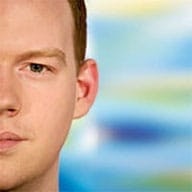
Browser Support
The View Transition API makes it easy to change the DOM in a single step, while creating an animated transition between the two states. It's available in Chrome 111+.
Why do we need this feature?
Page transitions not only look great, they also communicate direction of flow, and make it clear which elements are related from page to page. They can even happen during data fetching, leading to a faster perception of performance.
But, we already have animation tools on the web, such as CSS transitions , CSS animations , and the Web Animation API , so why do we need a new thing to move stuff around?
The truth is, state transitions are hard, even with the tools we already have.
Even something like a simple cross-fade involves both states being present at the same time. That presents usability challenges, such as handling additional interaction on the outgoing element. Also, for users of assistive devices, there's a period where both the before and after state are in the DOM at the same time, and things may move around the tree in a way that's fine visually, but can easily cause reading position and focus to be lost.
Handling state changes is particularly challenging if the two states differ in scroll position. And, if an element is moving from one container to another, you can run into difficulties with overflow: hidden and other forms of clipping, meaning you have to restructure your CSS to get the effect you want.
It isn't impossible, it's just really hard .
View Transitions give you an easier way, by allowing you to make your DOM change without any overlap between states, but create a transition animation between the states using snapshotted views.
Additionally, although the current implementation targets single page apps (SPAs), this feature will be expanded to allow for transitions between full page loads, which is currently impossible.
Standardization status
The feature is being developed within the W3C CSS Working Group as a draft specification .
Once we're happy with the API design, we'll start the processes and checks required to ship this feature to stable.
Developer feedback is really important, so please file issues on GitHub with suggestions and questions.
The simplest transition: A cross-fade
The default View Transition is a cross-fade, so it serves as a nice introduction to the API:
Where updateTheDOMSomehow changes the DOM to the new state. That can be done however you want: Add/removing elements, changing class names, changing styles… it doesn't matter.
And just like that, pages cross-fade:
Ok, a cross-fade isn't that impressive. Thankfully, transitions can be customized, but before we get to that, we need to understand how this basic cross-fade worked.
How these transitions work
Taking the code sample from above:
When .startViewTransition() is called, the API captures the current state of the page. This includes taking a screenshot.
Once that's complete, the callback passed to .startViewTransition() is called. That's where the DOM is changed. Then, the API captures the new state of the page.
Once the state is captured, the API constructs a pseudo-element tree like this:
The ::view-transition sits in an overlay, over everything else on the page. This is useful if you want to set a background color for the transition.
::view-transition-old(root) is a screenshot of the old view, and ::view-transition-new(root) is a live representation of the new view. Both render as CSS 'replaced content' (like an <img> ).
The old view animates from opacity: 1 to opacity: 0 , while the new view animates from opacity: 0 to opacity: 1 , creating a cross-fade.
All of the animation is performed using CSS animations, so they can be customized with CSS.
Simple customization
All of the pseudo-elements above can be targeted with CSS, and since the animations are defined using CSS, you can modify them using existing CSS animation properties. For example:
With that one change, the fade is now really slow:
Ok, that's still not impressive. Instead, let's implement Material Design's shared axis transition :
And here's the result:
Transitioning multiple elements
In the previous demo, the whole page is involved in the shared axis transition. That works for most of the page, but it doesn't seem quite right for the heading, as it slides out just to slide back in again.
To avoid this, you can extract the header from the rest of the page so it can be animated separately. This is done by assigning a view-transition-name to the element.
The value of view-transition-name can be whatever you want (except for none , which means there's no transition name). It's used to uniquely identify the element across the transition.
And the result of that:
Now the header stays in place and cross-fades.
That CSS declaration caused the pseudo-element tree to change:
There are now two transition groups. One for the header, and another for the rest. These can be targeted independently with CSS, and given different transitions. Although, in this case main-header was left with the default transition, which is a cross-fade.
Well, ok, the default transition isn't just a cross fade, the ::view-transition-group also transitions:
- Position and transform (via a transform )
That hasn't mattered until now, as the header is the same size and position both sides of the DOM change. But we can also extract the text in the header:
fit-content is used so the element is the size of the text, rather than stretching to the remaining width. Without this, the back arrow reduces the size of the header text element, whereas we want it to be the same size in both pages.
So now we have three parts to play with:
But again, just going with the defaults:
Now the heading text does a little satisfying slide across to make space for the back button.
Debugging transitions
Since View Transitions are built on top of CSS animations, the Animations panel in Chrome DevTools is great for debugging transitions.
Using the Animations panel, you can pause the next animation, then scrub back and forth through the animation. During this, the transition pseudo-elements can be found in the Elements panel.
Transitioning elements don't need to be the same DOM element
So far we've used view-transition-name to create separate transition elements for the header, and the text in the header. These are conceptually the same element before and after the DOM change, but you can create transitions where that isn't the case.
For instance, the main video embed can be given a view-transition-name :
Then, when the thumbnail is clicked, it can be given the same view-transition-name , just for the duration of the transition:
And the result:
The thumbnail now transitions into the main image. Even though they're conceptually (and literally) different elements, the transition API treats them as the same thing because they shared the same view-transition-name .
The real code for this is a little more complicated than the simple example above, as it also handles the transition back to the thumbnail page. See the source for the full implementation.
Custom entry and exit transitions
Look at this example:
The sidebar is part of the transition:
But, unlike the header in the previous example, the sidebar doesn't appear on all pages. If both states have the sidebar, the transition pseudo-elements look like this:
However, if the sidebar is only on the new page, the ::view-transition-old(sidebar) pseudo-element won't be there. Since there's no 'old' image for the sidebar, the image-pair will only have a ::view-transition-new(sidebar) . Similarly, if the sidebar is only on the old page, the image-pair will only have a ::view-transition-old(sidebar) .
In the demo above, the sidebar transitions differently depending on whether it's entering, exiting, or present in both states. It enters by sliding from the right and fading in, it exits by sliding to the right and fading out, and it stays in place when it's present in both states.
To create specific entry and exit transitions, you can use the :only-child pseudo-class to target the old/new pseudo-element when it's the only child in the image-pair:
In this case, there's no specific transition for when the sidebar is present in both states, since the default is perfect.
Async DOM updates, and waiting for content
The callback passed to .startViewTransition() can return a promise, which allows for async DOM updates, and waiting for important content to be ready.
The transition won't be started until the promise fulfills. During this time, the page is frozen, so delays here should be kept to a minimum. Specifically, network fetches should be done before calling .startViewTransition() , while the page is still fully interactive, rather than doing them as part of the .startViewTransition() callback.
If you decide to wait for images or fonts to be ready, be sure to use an aggressive timeout:
However, in some cases it's better to avoid the delay altogether, and use the content you already have.
Making the most of content you already have
In the case where the thumbnail transitions to a larger image:
The default transition is to cross-fade, which means the thumbnail could be cross-fading with a not-yet-loaded full image.
One way to handle this is to wait for the full image to load before starting the transition. Ideally this would be done before calling .startViewTransition() , so the page remains interactive, and a spinner can be shown to indicate to the user that things are loading. But in this case there's a better way:
Now the thumbnail doesn't fade away, it just sits underneath the full image. This means if the new view hasn't loaded, the thumbnail is visible throughout the transition. This means the transition can start straight away, and the full image can load in its own time.
This wouldn't work if the new view featured transparency, but in this case we know it doesn't, so we can make this optimization.
Handling changes in aspect ratio
Conveniently, all the transitions so far have been to elements with the same aspect ratio, but that won't always be the case. What if the thumbnail is 1:1, and the main image is 16:9?
In the default transition, the group animates from the before size to the after size. The old and new views are 100% width of the group, and auto height, meaning they keep their aspect ratio regardless of the group's size.
This is a good default, but it isn't what we want in this case. So:
This means the thumbnail stays in the center of the element as the width expands, but the full image 'un-crops' as it transitions from 1:1 to 16:9.
Changing the transition depending on device state
You may want to use different transitions on mobile vs desktop, such as this example which performs a full slide from the side on mobile, but a more subtle slide on desktop:
This can be achieved using regular media queries:
You may also want to change which elements you assign a view-transition-name depending on matching media queries.
Reacting to the 'reduced motion' preference
Users can indicate they prefer reduced motion via their operating system, and that preference is exposed via CSS .
You could chose to prevent any transitions for these users:
However, a preference for 'reduced motion' doesn't mean the user wants no motion . Instead of the above, you could choose a more subtle animation, but one that still expresses the relationship between elements, and the flow of data.
Changing the transition depending on the type of navigation
Sometimes a navigation from one particular type of page to another should have a specifically tailored transition. Or, a 'back' navigation should be different to a 'forward' navigation.
The best way to handle these cases is to set a class name on <html> , also known as the document element:
This example uses transition.finished , a promise that resolves once the transition has reached its end state. Other properties of this object are covered in the API reference .
Now you can use that class name in your CSS to change the transition:
As with media queries, the presence of these classes could also be used to change which elements get a view-transition-name .
Transitioning without freezing other animations
Take a look at this demo of a video transitioning position:
Did you see anything wrong with it? Don't worry if you didn't. Here it is slowed right down:
During the transition, the video appears to freeze, then the playing version of the video fades in. This is because the ::view-transition-old(video) is a screenshot of the old view, whereas the ::view-transition-new(video) is a live image of the new view.
You can fix this, but first, ask yourself if it's worth fixing. If you didn't see the 'problem' when the transition was playing at its normal speed, I wouldn't bother changing it.
If you really want to fix it, then don't show the ::view-transition-old(video) ; switch straight to the ::view-transition-new(video) . You can do this by overriding the default styles and animations:
And that's it!
Now the video plays throughout the transition.
Animating with JavaScript
So far, all the transitions have been defined using CSS, but sometimes CSS isn't enough:
A couple of parts of this transition can't be achieved with CSS alone:
- The animation starts from the click location.
- The animation ends with the circle having a radius to the farthest corner. Although, hopefully this will be possible with CSS in future .
Thankfully, you can create transitions using the Web Animation API !
This example uses transition.ready , a promise that resolves once the transition pseudo-elements have been successfully created. Other properties of this object are covered in the API reference .
Transitions as an enhancement
The View Transition API is designed to 'wrap' a DOM change and create a transition for it. However, the transition should be treated as an enhancement, as in, your app shouldn't enter an 'error' state if the DOM change succeeds, but the transition fails. Ideally the transition shouldn't fail, but if it does, it shouldn't break the rest of the user experience.
In order to treat transitions as an enhancement, take care not to use transition promises in a way that would cause your app to throw if the transition fails.
The problem with this example is that switchView() will reject if the transition cannot reach a ready state, but that doesn't mean that the view failed to switch. The DOM may have successfully updated, but there were duplicate view-transition-name s, so the transition was skipped.
This example uses transition.updateCallbackDone to wait for the DOM update, and to reject if it fails. switchView no longer rejects if the transition fails, it resolves when the DOM update completes, and rejects if it fails.
If you want switchView to resolve when the new view has 'settled', as in, any animated transition has completed or skipped to the end, replace transition.updateCallbackDone with transition.finished .
Not a polyfill, but…
I don't think this feature can be polyfilled in any useful way, but I'm happy to be proven wrong!
However, this helper function makes things much easier in browsers that don't support view transitions:
And it can be used like this:
In browsers that don't support View Transitions, updateDOM will still be called, but there won't be an animated transition.
You can also provide some classNames to add to <html> during the transition, making it easier to change the transition depending on the type of navigation .
You can also pass true to skipTransition if you don't want an animation, even in browsers that support View Transitions. This is useful if your site has a user preference to disable transitions.
Working with frameworks
If you're working with a library or framework that abstracts away DOM changes, the tricky part is knowing when the DOM change is complete. Here's a set of examples, using the helper above , in various frameworks.
- React —the key here is flushSync , which applies a set of state changes synchronously. Yes, there's a big warning about using that API, but Dan Abramov assures me it's appropriate in this case. As usual with React and async code, when using the various promises returned by startViewTransition , take care that your code is running with the correct state.
- Vue.js —the key here is nextTick , which fulfills once the DOM has been updated.
- Svelte —very similar to Vue, but the method to await the next change is tick .
- Lit —the key here is the this.updateComplete promise within components, which fulfills once the DOM has been updated.
- Angular —the key here is applicationRef.tick , which flushes pending DOM changes. As of Angular version 17 you can use withViewTransitions that comes with @angular/router .
API reference
Start a new ViewTransition .
updateCallback is called once the current state of the document is captured.
Then, when the promise returned by updateCallback fulfills, the transition begins in the next frame. If the promise returned by updateCallback rejects, the transition is abandoned.
Instance members of ViewTransition :
A promise that fulfills when the promise returned by updateCallback fulfills, or rejects when it rejects.
The View Transition API wraps a DOM change and creates a transition. However, sometimes you don't care about the success/failure of the transition animation, you just want to know if and when the DOM change happens. updateCallbackDone is for that use-case.
A promise that fulfills once the pseudo-elements for the transition are created, and the animation is about to start.
It rejects if the transition cannot begin. This can be due to misconfiguration, such as duplicate view-transition-name s, or if updateCallback returns a rejected promise.
This is useful for animating the transition pseudo-elements with JavaScript .
A promise that fulfills once the end state is fully visible and interactive to the user.
It only rejects if updateCallback returns a rejected promise, as this indicates the end state wasn't created.
Otherwise, if a transition fails to begin, or is skipped during the transition, the end state is still reached, so finished fulfills.
Skip the animation part of the transition.
This won't skip calling updateCallback , as the DOM change is separate to the transition.
Default style and transition reference
Absolutely positioned.
Transitions width and height between the 'before' and 'after' states.
Transitions transform between the 'before' and 'after' viewport-space quad.
Absolutely positioned to fill the group.
Has isolation: isolate to limit the effect of the plus-lighter blend mode on the old and new views.
Absolutely positioned to the top-left of the wrapper.
Fills 100% of the group width, but has an auto height, so it will maintain its aspect ratio rather than filling the group.
Has mix-blend-mode: plus-lighter to allow for a true cross-fade.
The old view transitions from opacity: 1 to opacity: 0 . The new view transitions from opacity: 0 to opacity: 1 .
Developer feedback is really important at this stage, so please file issues on GitHub with suggestions and questions.
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2021-08-17 UTC.
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
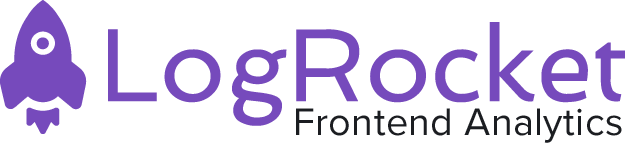
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Getting started with the View Transitions API
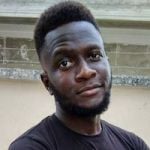
The web sets no limitations or restrictions on the channels through which we can present and display content or the level of interactivity and unique experiences we can integrate into our applications.
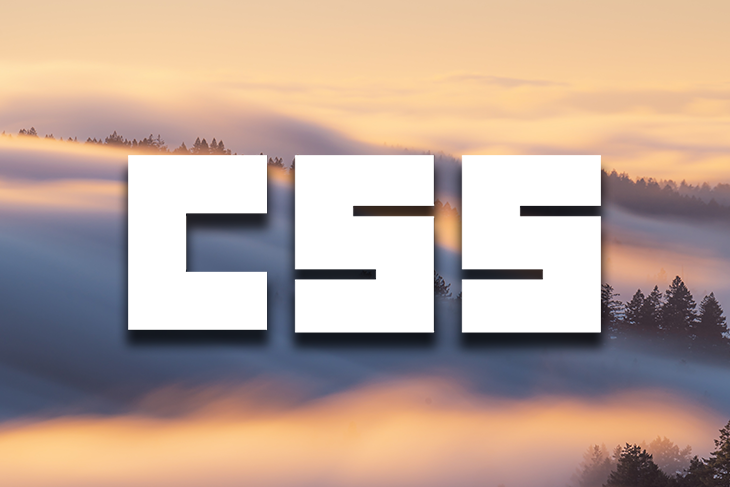
As developers, we can define how users experience the web by utilizing the many tools and technologies available, ensuring that users experience the uniqueness and beauty of the web.
One common way of spicing up the web is adding animations and transitions to applications, like a background color that changes when a user hovers over a button or blog cards sliding in from the left and right as a user scrolls down a page. These simple transitions can distinguish between a plain experience and a vibrant one.
Beyond using third-party tools like Animate.css , Animsta , GSAP , or plain old CSS , we can leverage a browser API called the View Transition API to create stunning and eye-catching animations and transitions. In this article, we will learn how the API works and explore a demo.
Jump ahead:
What is the View Transitions API?
How the view transitions api works, setting up the javascript aspect.
The View Transitions API , formally known as the Shared Element Transitions API, is an API for creating transitions and animations in single-page animations (SPA) with ease and minimal effort. It is restricted to SPAs because those are the only application it currently supports.
The API gives us control over how to define animations and transitions. We can use the default animation properties supported by browsers or create custom effects .
Also, the API automatically transitions the size and position of an element for us. It tracks and calculates the changes between the before and after states of these properties and adds appropriate transitions to them.
At the time of writing, the View Transitions API is only available in Chrome v104+ and Canary. To activate it, first, navigate to chrome://flags/ . Then, enable the Experimental Web Platform features and viewTransition API for navigations .
The magic of how the View Transitions API works happens behind the scenes. The browser takes a screenshot of the current state of a page and handles the transition as the page shifts to another state. The state change could be an element moving from one position to another or page navigation, among others.
The browser updates the DOM and applies the necessary logic needed to animate between states. Then, the browser applies a cross-fade as the default transition between the previous and current states.
When the API is active, it constructs a pseudo-element tree like this:
Let’s breakdown the pseudo-elements in the code snippet:
- ::view-transition : The container element for other pseudo-elements
- ::view-transition-group : Responsible for animating the size and position between the two states
- ::view-transition-image-pair : Holds the screenshot of the old and new states
- ::view-transition-old(root) : Holds the screenshot of the previous state
- ::view-transition-new(root) : Holds the representation of the new state
The previous state animates from opacity: 1 to opacity: 0 , while the new state goes from opacity: 0 to opacity: 1 , creating the cross-fade. The animation is done with CSS animations , meaning we can customize them with CSS:
We can use the default cross-fade transition or set up even more complex ones that fit our taste.
A great thing about this API is that the browser does all the heavy lifting of tracking and calculating the changes between states and handles the transitions in a highly performant manner.
View Transitions API demo
Let’s create a simple demo app to see how the View Transitions API works in practice. We’ll create this demo with basic HTML , CSS , and JavaScript .
It’s a to-do app that consists of two actions of tasks: Not Done and Done . The tasks that are undone come with a clickable button to mark them as done.
The GIF below shows the UI and functionality of the app. Take note of how a transition effect takes place as the tasks move from one section to another:
Here’s a snapshot of the HTML:
In the code above, we pass an isTaskComplete function to the click events of the buttons. We’ll learn more about what isTaskComplete does next.
Now, let’s set up the JavaScript aspect of the demo and explore what the isTaskComplete function does:
Let’s break down the code above. First, the isTaskComplete function takes in a Boolean as an argument. From there, we initialized a task variable that contains the li closest to where the event occurred.
The value of the destination variable changes based on the state of the taskComplete . When taskComplete is true , the destination is list-done . However, when taskComplete is false , the destination is list-not-done .
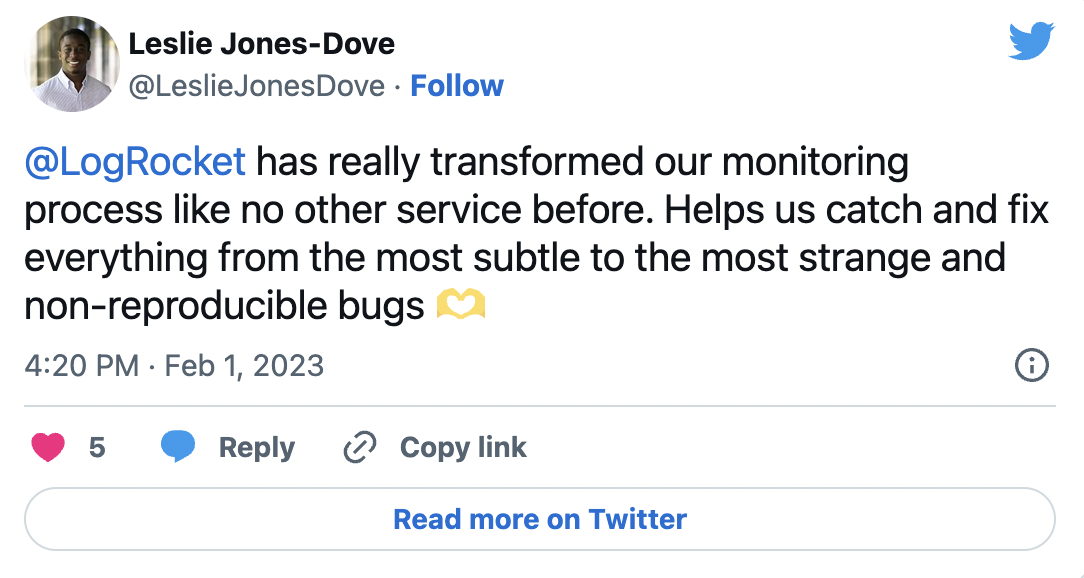
Over 200k developers use LogRocket to create better digital experiences

We also added a task-done class to the task to hide the button when a task is marked as completed. In addition, we checked if the browser supports the View Transitions API with the if statement. If it doesn’t, we create a taskTransition instance using the createDocumentTransition method to trigger the transition using the start method.
If the browser doesn’t support the View Transitions API, we append the task directly without adding any transition to the change in state from one position to another.
Here’s the task-done class we added. It is responsible for hiding a task’s button :
This implementation sheds some light on how the View Transitions API works.
The View Transitions API opens up a new frontier of possibilities regarding the unique and pleasing experiences we can bring to the web. As great as it is, it is important to remember that, as of this writing, it is still in beta. So, the API is subject to change at any moment. However, that won’t stop us from experimenting and learning about it!
Here are some great examples, demos, and implementations of the View Transitions API that you should check out: Geoff Rich built this fruit data app with SvelteKit and wrote an article about it, Maxi Ferreira made a movie app with Astro and explained how he achieved this, and Jake Archibald created a video playlist app , and there’s a video on YouTube.
Is your frontend hogging your users' CPU?
As web frontends get increasingly complex, resource-greedy features demand more and more from the browser. If you’re interested in monitoring and tracking client-side CPU usage, memory usage, and more for all of your users in production, try LogRocket .
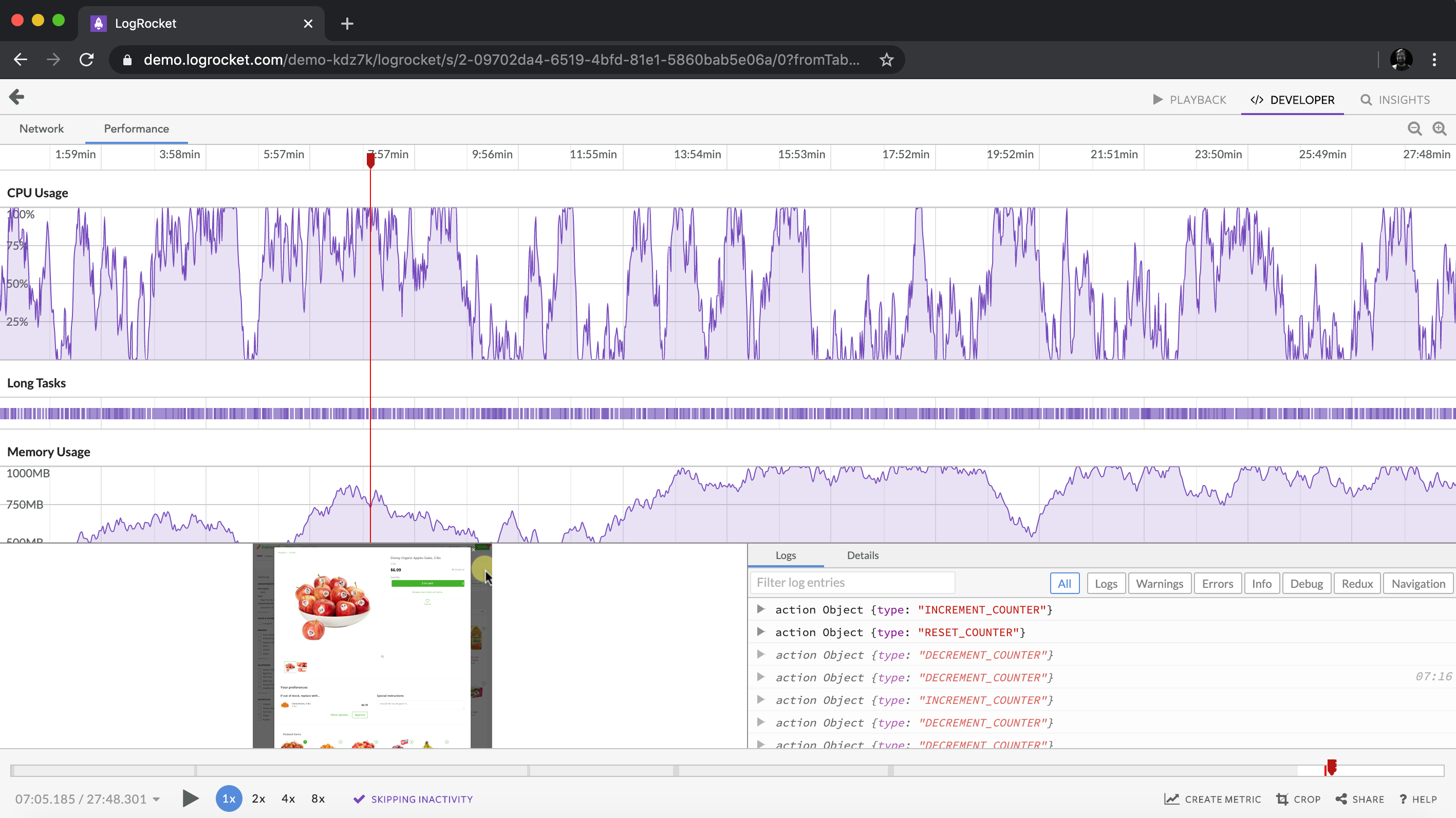
LogRocket is like a DVR for web and mobile apps, recording everything that happens in your web app, mobile app, or website. Instead of guessing why problems happen, you can aggregate and report on key frontend performance metrics, replay user sessions along with application state, log network requests, and automatically surface all errors.
Modernize how you debug web and mobile apps — start monitoring for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #vanilla javascript
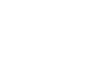
Stop guessing about your digital experience with LogRocket
Recent posts:.
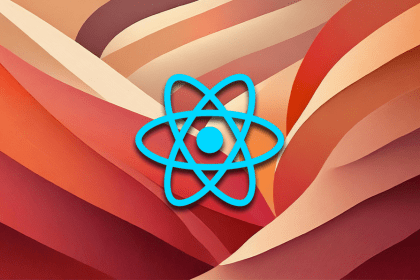
Comparing React state tools: Mutative vs. Immer vs. reducers
Mutative processes data with better performance than both Immer and native reducers. Let’s compare these data handling options in React.
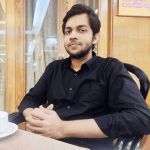
Radix UI adoption guide: Overview, examples, and alternatives
Radix UI is quickly rising in popularity and has become an excellent go-to solution for building modern design systems and websites.
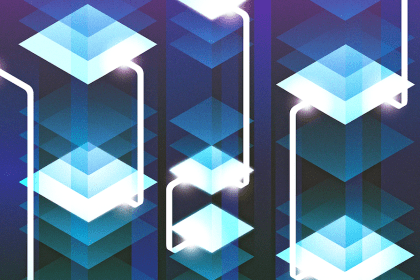
Understanding the CSS revert-layer keyword
In this article, we’ll explore CSS cascade layers — and, specifically, the revert-layer keyword — to help you refine your styling strategy.
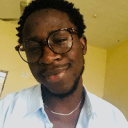
Exploring Nushell, a Rust-powered, cross-platform shell
Nushell is a modern, performant, extensible shell built with Rust. Explore its pros, cons, and how to install and get started with it.
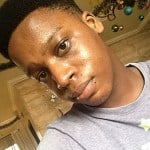
Leave a Reply Cancel reply
An Introduction to the View Transitions API
Share this article
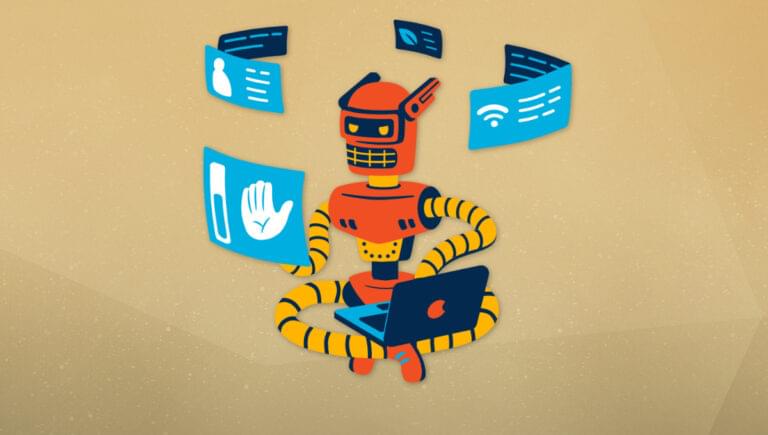
New Old Techniques
Creating in-page transitions, asynchronous dom updates, creating more sophisticated transitions, using the web animations api, creating multi-page navigation transitions, disabling animations, frequently asked questions (faqs) about view transitions api.
The new View Transitions API offers an easier way to animate between two DOM states — even between page loads. It’s a progressive enhancement that works today.
CSS transitions and animations have revolutionized web effects over the past decade, but not everything is easy. Consider a list of elements — such as ten images with titles — which we want to transition into a new list of elements using a cross-fade. The current approach:
- retain the old DOM elements
- build the new DOM elements, append them to the page, ensuring they’re in an appropriate location
- fade out the old set while fading in the new set, then
- (optionally) replace the old DOM elements with the new
It’s not been possible to simply update the DOM — until now! The View Transitions API uses the following process:
- The API takes a snapshot of the current page state.
- We update the DOM adding or removing elements as necessary.
- The API takes a snapshot of the new page state.
- The API animates between the two states, using a default fade or whatever CSS animations we define.
We only need to update the DOM as we’re already doing. A few lines of additional code can progressively enhance the page when the View Transitions API is available to create presentation-like effects.
The API is experimental, but recent Chromium-based browsers support in-page, single-document DOM-based effects.
A viewTransition API for navigations is also available in Chrome 115+ and offers animations between individual page loads — such as on typical WordPress sites. This is even easier to use and requires no JavaScript.
Mozilla and Apple haven’t revealed their intentions for implementing the API in Firefox and Safari. Any browser without the View Transitions API will continue to work, so it’s safe to add effects today.
Developers of a certain age may be experiencing déjà vu. Microsoft added element and whole page transitions in Internet Explorer 4.0 (released in 1997) with further updates in IE5.5 (released in 2000). We could add PowerPoint-inspired boxes, circles, wipes, dissolves, blinds, slides, strips, and spirals with a <meta> tag:
Strangely, the technique never became widely adopted. It wasn’t a web standard, but the W3C was in its infancy — and developers were happy to use plenty of other IE-specific technologies!
Why has it taken a quarter of a century for an alternative to appear?!
View the following CodePen example in Chrome and click the navigation in the header to see a one-second fade between the two states.
See the Pen Transitions API example 1 by SitePoint ( @SitePoint ) on CodePen .
The HTML page has two <article> elements with the IDs article1 and article2 for the blocks of content:
A switchArticle() function handles all DOM updates. It shows or hides each article by adding or removing a hidden attribute. On page load, the active article is determined from the page URL’s location.hash or, if that’s not set, the first <article> element:
An event handler function monitors all page clicks and calls switchArticle() when the user clicks a link with a #hash :
We can now update this handler to use View Transitions by passing the switchArticle() function as a callback to document.startViewTransition() (checking the API is available first):
document.startViewTransition() takes a snapshot of the initial state, runs switchArticle() , takes a new snapshot of the new state, and creates a default half-second fade between the two.
The following selectors are available in CSS to target the old and new states:
The example above increases the animation duration to one second so the fade effect is more noticeable:
A view-transition-group(root) can apply effects to both old and new states at the same time, although we’re unlikely to apply the same animation in most cases.
The callback passed to document.startViewTransition() can return a promise so asynchronous updates are possible. For example:
This freezes the page until the promise fulfills, so delays could impact the user experience. It’s more efficient to run as much code as possible outside the call to .startViewTransition() . For example:
The following CodePen demo adds a nicer animation using the ::view-transition-old(root) and ::view-transition-new(root) selectors.
See the Pen Transitions API example 2 by SitePoint ( @SitePoint ) on CodePen .
The CSS defines transition-out and transition-in animations with fading and rotation:
The animations apply to the whole page — including the <header> element, which looks a little strange. We can apply animations (or no animation) to individual elements by setting a view-transition-name :
We can now target that element and apply a different animation:
In this case, we don’t want the header to have any effects, so it’s not necessary to define any animation. The ::view-transition-old(root) and ::view-transition-new(root) selectors now apply to all elements except for the <header> . It remains in-place.
See the Pen Transitions API example 3 by SitePoint ( @SitePoint ) on CodePen .
Because we’re defining effects in CSS, we can use developer tool features such as the Animations panel to examine and debug our animations in more detail.
While CSS is enough for most effects, the Web Animations API permits further timing and effect control in JavaScript.
document.startViewTransition() returns an object that runs a .ready promise which resolves when the transition old and new pseudo-elements are available (note the pseudoElement property in the second .animate() parameter):
We can also use View Transitions as the user navigates between page loads on multi-page applications (MPA) such as typical WordPress sites. It’s known as the viewTransition API for navigations , which we must enable in chrome://flags/ in Chrome 115 (currently the Canary nightly build for developers ). The flag is also available in previous releases of the browser, but the API may be missing or unstable.
The process is easier than in-page transitions because it’s enabled with a single meta tag in the HTML <head> :
We can then define ::view-transition-old and ::view-transition-new CSS selectors in an identical way to those shown above. We don’t require any JavaScript unless we want to use the Web Animations API .
The navigations API may or may not be enabled by default when Chrome 115 final is released. We can use the technique today because browsers that don’t support the API will fall back to standard, non-animated page loads.
Animations can trigger discomfort for some people with motion disorders. Most operating systems provide a user preference setting to disable effects. We can detect this with the CSS prefers-reduced-motion media query and switch off animations accordingly:
The View Transitions API simplifies animations when altering element states in-page and between page loads. Transitions of this type were possible before, but they required a considerable amount of JavaScript — and we had to be careful not to break browser navigation such as the back button.
The API is new. There’s no guarantee it will remain unchanged, become a W3C standard, or have implementations in Firefox and Safari. However, we can use the API today because it’s a progressive enhancement. Our applications will continue to work in browsers which don’t support the API; they just won’t show animations. There’s a risk certainty the API will change but, even if we have to do some maintenance, our old code shouldn’t break the site and updates are likely to be minimal.
The downside? The API could lead to an explosion of annoyingly long and wild animations across the Web because site owners consider them to be “on-brand”! Ideally, animations should be fast and subtle to highlight a UI change. Less is often more.
Further references:
- Chrome Developers: Smooth and simple transitions with the View Transitions API : lots of examples, suggestions, and techniques
- MDN: View Transitions API
- W3C: CSS View Transitions Module Level 1
What is the View Transitions API and how does it work?
The View Transitions API is a web-based interface that allows developers to create smooth, seamless transitions between different views or states of a web application. It works by providing a set of methods and properties that developers can use to define and control the behavior of transitions. This includes the ability to specify the duration, timing function, and delay of a transition, as well as the ability to pause, resume, or cancel a transition in progress.
How can I use the View Transitions API in my web application?
To use the View Transitions API in your web application, you first need to include it in your project. This can be done by adding a reference to the API in your HTML file. Once the API is included, you can use its methods and properties to define and control transitions. For example, you can use the transition property to specify the duration, timing function, and delay of a transition, and the pause , resume , and cancel methods to control a transition in progress.
What are the benefits of using the View Transitions API?
The View Transitions API offers several benefits for web developers. First, it allows for the creation of smooth, seamless transitions between different views or states of a web application, which can enhance the user experience. Second, it provides a high level of control over the behavior of transitions, allowing developers to fine-tune the appearance and timing of transitions to suit their needs. Finally, the API is web-based, meaning it can be used in any web application, regardless of the underlying technology stack.
Are there any limitations or drawbacks to using the View Transitions API?
While the View Transitions API is a powerful tool for creating transitions, it does have some limitations. For example, it may not be supported by all browsers, particularly older ones. Additionally, while the API provides a high level of control over transitions, it can also be complex to use, particularly for developers who are not familiar with its methods and properties. Finally, like any API, it can be subject to changes and updates, which may require developers to update their code to maintain compatibility.
Can I use the View Transitions API with other web technologies?
Yes, the View Transitions API can be used in conjunction with other web technologies, such as HTML, CSS, and JavaScript. In fact, it is designed to be used as part of a larger web development toolkit, complementing and enhancing the capabilities of these other technologies. For example, you can use the API to create transitions that are triggered by JavaScript events, or to animate changes to CSS properties.
How can I learn more about the View Transitions API?
There are several resources available for learning more about the View Transitions API. These include the official API documentation, which provides a comprehensive overview of the API’s methods and properties, as well as tutorials and examples that demonstrate how to use the API in practice. Additionally, there are numerous online communities and forums where developers can share tips and tricks, ask questions, and get help with using the API.
What are some examples of applications that use the View Transitions API?
The View Transitions API is used in a wide range of web applications, from simple websites to complex web-based applications. For example, it can be used to create transitions between different pages of a website, or between different states of a web-based game or interactive application. It can also be used to animate changes to the layout or appearance of a web page, such as expanding or collapsing a menu, or changing the color of a button.
How can I troubleshoot issues with the View Transitions API?
If you’re experiencing issues with the View Transitions API, there are several steps you can take to troubleshoot the problem. First, check the API documentation to ensure you’re using the methods and properties correctly. Second, use a web development tool such as Chrome DevTools to inspect your code and identify any errors. Finally, consider reaching out to the online developer community for help. Many developers are willing to share their expertise and may be able to provide insights or solutions to your problem.
Can I use the View Transitions API on mobile devices?
Yes, the View Transitions API is designed to be used on both desktop and mobile devices. However, keep in mind that the performance and behavior of transitions may vary depending on the device and browser. It’s always a good idea to test your transitions on multiple devices and browsers to ensure they work as expected.
Is the View Transitions API free to use?
Yes, the View Transitions API is a free, open-source technology. This means you can use it in your projects without having to pay any licensing fees. However, as with any open-source technology, it’s important to understand and comply with the terms of the API’s license, which may require you to share any modifications you make to the API or to attribute the original creators.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler .
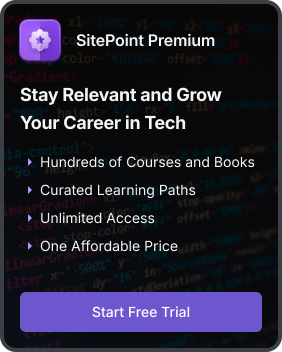
View Transitions API & meta frameworks: a practical guide
August 30, 2023 - 16 min to read
No more boring page reloads in MPAs. The View Transitions API makes website navigation smoother, like in mobile apps or SPAs. Plus, This API removes a lot of the complex JavaScript SPAs needed for these transitions.
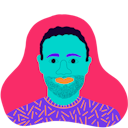
By Mojtaba Seyedi
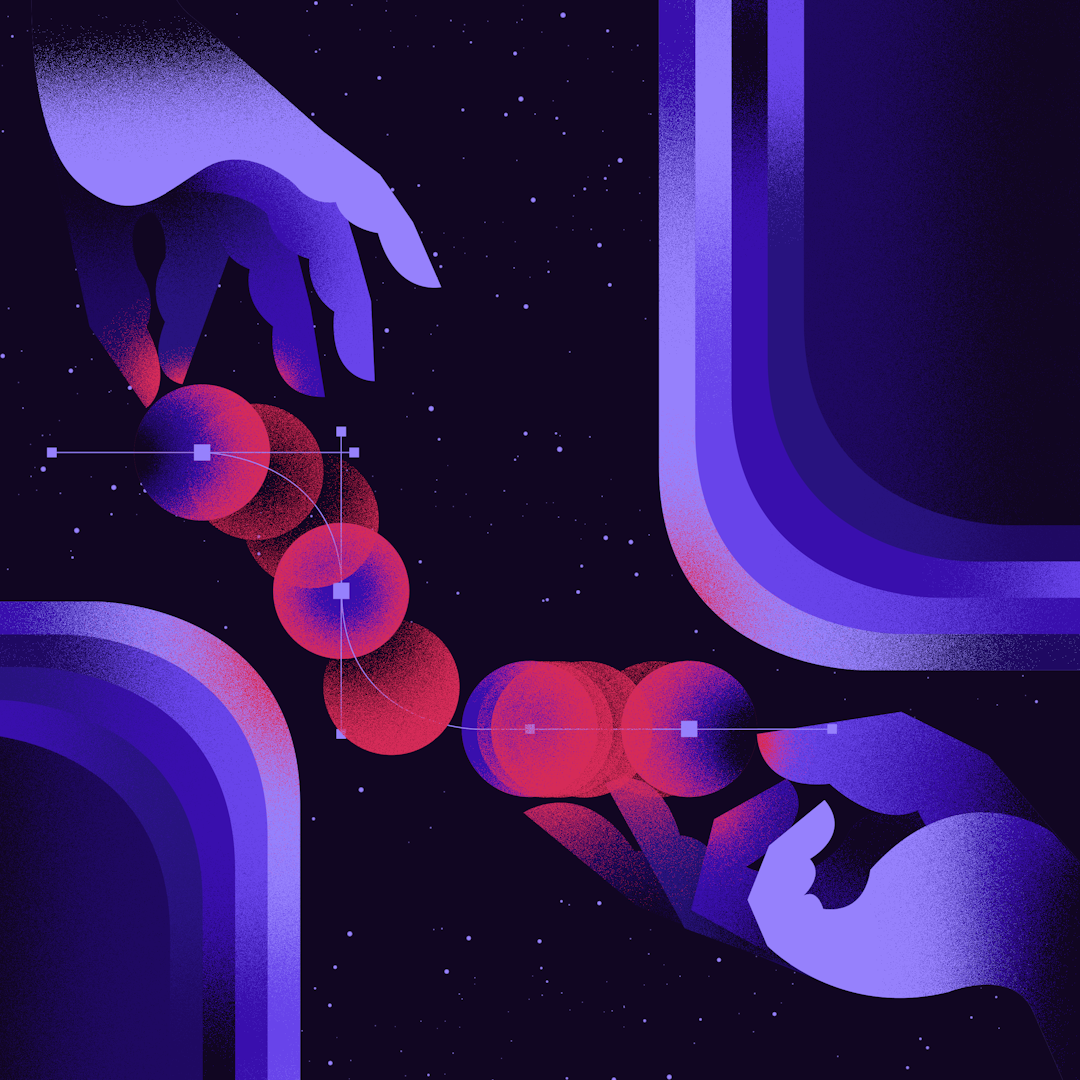
Smooth animations during navigation within a mobile app have been around for some time. However, achieving the same effect on the web has been a challenge, often requiring complex and unique approaches—until now. Enter the View Transitions API, a simple and straightforward browser-based solution.
In this article, I'll explain what this API is and how it works. We will learn to create smooth and simple transitions between states and pages. We will also explore how the View Transitions API pairs up with meta frameworks like Astro and Nuxt.
Get ready for a fun and exciting ride with transitions.
What is the View Transitions API?
View transition examples, create your first view transition, how view transitions work behind the scenes, spa view transitions, mpa view transitions, customizing view transitions with css animations, named view transitions, view transition and accessibility, enhancing with javascript when css isn’t enough, how to disable view transitions, view transition "gotcha", view transition in astro, view transition in nuxt, view transition in sveltekit, browser supports.
The View Transitions API allows you to add animated transitions between two states of visual DOM changes. These changes could range from something as small as adding a new element to the DOM, to bigger changes, such as navigating from one page to another.
To illustrate this, consider the following scenarios:
Adding elements with view transitions
In the following video, clicking buttons adds an <img> element to the page. One button employs the View Transitions API, while the other does not.
Navigating pages with view transitions
For bigger changes like page navigation, the View Transitions API truly shines. The following video shows the contrast between navigation with and without the API. I made the transition slower to highlight the effect.
The API is available in Chrome 111 and newer versions, so you can easily add it to your website using just a few lines of code as a progressive enhancement. This way, your site gets an upgrade, and you won't need to worry about browsers that don't support it yet.
This API was initially designed for Single-Page Applications (SPAs) but now it works also for Multi-Page Applications (MPAs).
Let's dive into some examples that'll get you excited about this API and help you understand its significance and possibilities. To try out these demos, make sure you're using the latest Chrome browser version and have enabled the viewTransition API for navigations flag.
Type chrome://flags in your browser's address bar, find the viewTransition API for navigations and change it to Enabled.
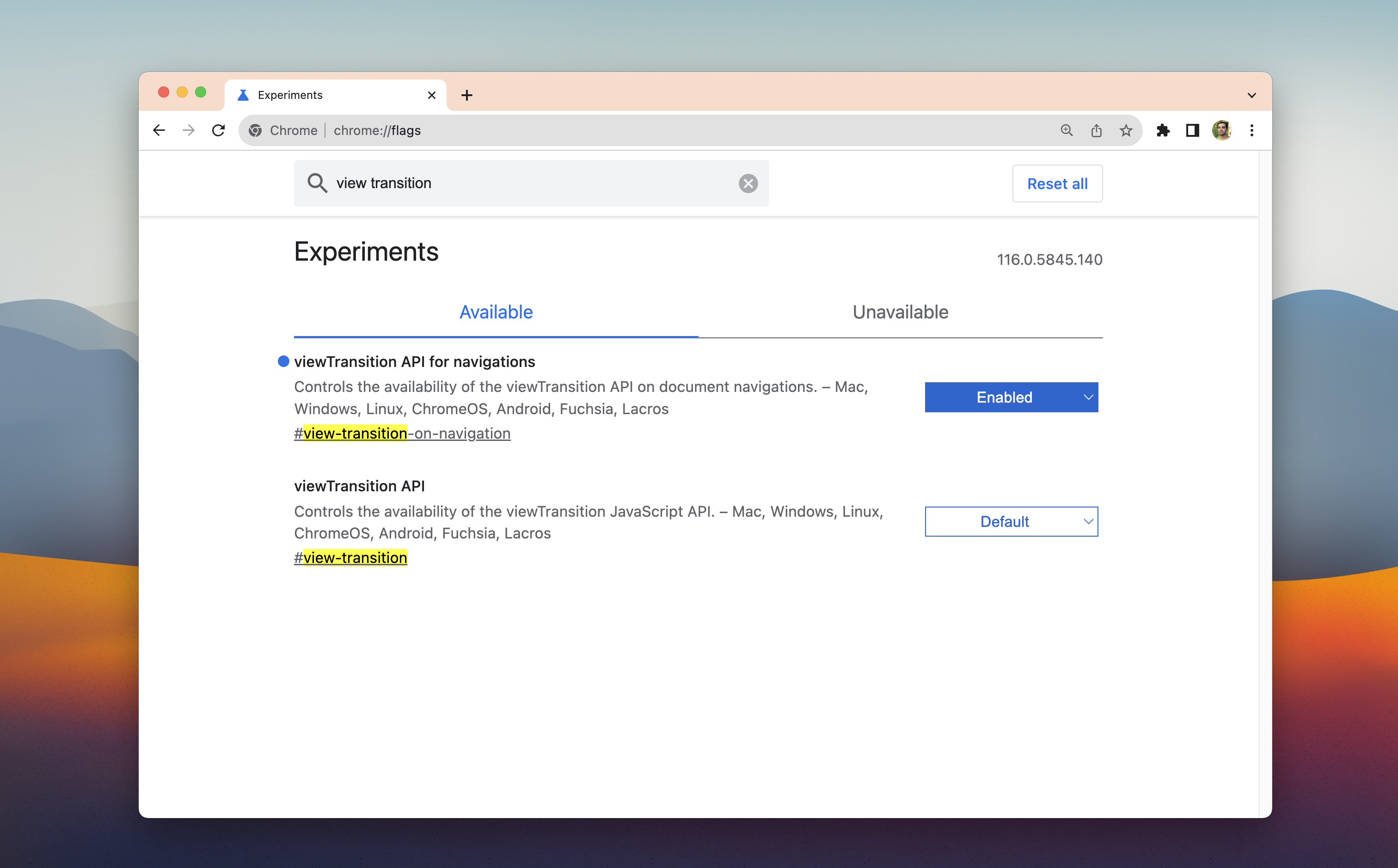
Let's start with two examples that illustrate the original intention behind the View Transitions API. This API was designed to introduce smooth transitions between different states in single-page applications.
These two demos have been crafted by Maxi Ferreira, and you can access the source code for both of them on GitHub. You'll find the link to the first demo's source code here , and you can interact with the demo directly through this link .
Here is the second demo:
Its source code is also on GitHub , and here is the live version if you want to give it a spin.
The previous demos were built using Astro . Now, let's explore the fact that you can achieve similar results even with websites that aren't built on any web frameworks. Here's a demo to showcase this:
Loading Video
You can play with the demo here .
Are you ready for something surprising? The demo you've just seen didn't use any JavaScript at all. It was a simple multi-page website built using HTML and CSS.
Now, here's the same demo, but this time with just a few lines of JavaScript added to enhance the transitions and make them more refined:
You can also find this demo here .
As you saw in the examples, the View Transition works great in route-level scenarios. However, I'd like to illustrate that you can also leverage this API at a more granular level, even down to an atomic scale—to create more exciting interactions.
For example, let's check out this demo made by Adam Argyle. You can see its code on CodePen .
He is using this API to add transitions to a drag-and-drop component.
Here's another neat example by Adam—a number counter .
There are more and more great examples, but I won't overwhelm you with them right now. I'm sure you're all set to learn how this API works and how to use it. Let's get started!
Before we get into how a view transition works, let's try something simple to see that it's actually quite straightforward.
Imagine we want to make an image appear on the page when we click a button. Normally, the image would just pop up without any fancy effects. Now, let's see how we can use this API to make the image transition smoothly.
Here is the HTML:
In JavaScript, let's add an <img> element to the page once this button is clicked:
Now, we only need to call the document.startViewTransition() method when we want to append the element to the page, like the following:
As you see in the demo below, you'll notice a smooth fade-in transition when you click the button.
Working with the View Transitions API is incredibly straightforward. However, before we create more examples, let’s take a moment to understand the underlying mechanics of how this works.
The View Transitions API might sound a bit mysterious, but once you understand how it works and create a few demos yourself, you get a good grasp of it. Now, let's peek behind the curtain to see what's happening.
When you trigger a view transition, like when you call a document.startViewTransition() , the browser takes a snapshot of the current page state. Think of this as a quick photo of what's on the screen right now.
Then, the magic begins. The callback function you provided in document.startViewTransition() gets called. This is where you can change things on the web page. The browser cleverly pauses rendering during this callback to prevent any flicker, and it does this very quickly.
Once your callback has done its thing, the browser takes another snapshot, but this time, it's of the new page state, the one you just changed. The browser uses these snapshots to create a special structure that's like an overlay on top of the page. This structure includes the old snapshot and the new one, stacked on top of each other.
This structure has different layers, like a tree of pseudo-elements. Each has its purpose, but we won't get into the details now. The important thing is that this overlay sits over everything else on the page.
The old snapshot ( ::view-transition-old ) and the live representation of the new state ( ::view-transition-new ) start their own special dance. The old image fades out (like turning down the opacity from 1 to 0), and the new image fades in (increasing the opacity from 0 to 1). This creates that familiar cross-fade effect.
Once this animation finishes, the overlay is removed, revealing the final page state underneath. It's cleverly done so that there's no time when both the old and new content exist together, which helps avoid issues with accessibility, usability, and layout.
Here's a visual that can make it easier for you to grasp these layers. Created by Bramus on Codepen :
Now comes the really neat part, the animation is controlled by CSS, and you can change how it looks.
For instance, you can make the cross-fade last longer like this:
And that's how the View Transitions API works its magic! It captures snapshots, applies your changes, animates them with a cross-fade, and presents the final result smoothly.
If you're curious about what each of those pseudo-elements does, here's a quick explanation for each:
- ::view-transition-group - animates size and position between the two states.
- ::view-transition-image-pair - provides blending isolation so the two images can correctly cross-fade.
- ::view-transition-old and ::view-transition-new - the visual states to cross-fade.
Using view transitions in a SPA is not very different than our simple demo in the previous example. In a single page application, you use JavaScript to dynamically change the DOM to the new state, which can be done by adding or removing elements, changing class names, changing styles, etc., but this all happens in a single function, so again you can wrap startViewTransition around that function.
Here's an example:
Now, the browser takes a look at the initial page, then gazes at this new page and animates between the two. After that, it moves us to the new page.
Most of the demos you've seen so far were transitions within the same document, a concept that was initially proposed and implemented in the browser. However, there's an expansion to this API called "Cross-document View Transitions," which allows you to add transitions while navigating across different documents. In other words, this means you can also add transitions to multi-page applications.
To see this in action, let's create a demo that we'll use throughout this article. I've put together a website consisting of several web pages, all without relying on any frameworks. These are just plain HTML and CSS. Here is the preview of the website here , and you can see the code on GitHub .
To enable the view transition between these pages, all you need to do is add the following meta tag to the HTML <head> of your document:
Let’s check out the demo .
Remember that at the time of writing, you need to use Chrome and enable the View Transitions API for navigations flag, as I explained at the beginning of the article.
Did you catch the smooth cross-fade effect in this demo? Pretty easy, isn't it?
This approach is known as the declarative way of adding view transitions. It works automatically and doesn't require any JavaScript. Just declaring the meta tag does the trick.
This isn't the end of the story. The View Transitions API allows you to customize your transitions and even create animations for page elements during navigation. Let's explore how to do that.
You might not always want the default cross-fade animation. Thankfully, the View Transitions API gives you the power to shape the animations the way you want, using the pseudo-elements in your CSS.
You'll be working with ::view-transition-old() for the state that's leaving and ::view-transition-new() for the state that's coming in.
Let's give our demo a makeover and switch the animation from a cross-fade to a sliding one:
Here is what our demo looks like now:
Here is the live version of the demo and the source code .
Now, what if we want to apply this magic to just a single part of our page, not the whole document?
Imagine you want an element on one page to morph smoothly into another element on a different page or state. To make this happen, you can use something called a named view transition.
The View Transitions API brings in a new tool called the view-transition-name property. This CSS property lets you assign the same special name to both of those elements. This name is what helps you create your named view transition.
This works for both SPA and MPA applications. Let's break it down by an example.
In this example, we have a small image on one page, and we want it to morph into a larger image on another page.
On the first page:
On the other page:
These two elements can either be the same element with different styles or even entirely different HTML elements. The important thing is that they share the same view-transition-name value, which triggers the view-transition effect.
Remember, you can pick any name you like, just not none . And keep those names unique per page. If two elements on the same page share the same view-transition-name value at the same time, the transition won't happen.
Do you remember the pseudo-elements tree we discussed? You create a new branch when you add another view transition using view-transition-name . Here's what the tree looks like now:
There is also a visualization for this made by Bramus on Codepen that can help:
This means we can customize the new view transition using the new pseudo-elements.
Now, let's apply view transitions to different elements in our demo. Up until now, we've used a single cross-fade animation for the entire page.
Let’s extract our banners from their pages so they can be animated separately. For our banners, we'll apply a slide-in animation effect.
Here is the result:
Remember, you don't always need to add view transitions to blend elements. Sometimes, you might only want to add view transitions to elements in the old or the new state, not in both. Think about the testimonials section on the home page or blockquote on the detail page.
Even though our blockquote is only present on a single page, we can add a view transition to animate them as the page loads.
You might have noticed that we're not using ::view-transition-old here. We're doing this because we want an animation when we arrive on the page. And when we leave the page, we've chosen not to include the sliding effect on purpose.
Thanks to the View Transitions API, we have the freedom to tailor our view transitions according to our preferences.
As shown in the following video, I've added a few additional view transitions to our demo.
You can view the final version of the demo here and explore its source code on GitHub .
When I look at the last demo myself, I feel like many animations are happening, and I don’t really like it. That's because design isn't my strong suit. This got me thinking about people with Vestibular disorder . No matter how cool an animation looks, it can be a problem for them. These users can tell their computer that they want less motion, and this choice is shown through CSS.
You can stop any transitions or pick a more subtle animation for these users using the prefers-reduced-motion media query.
Looking at our demo, what if we want to add a view transition to the thumbnail you see both on the home and detail page, like in the following video:
Sounds easy, right? We just need to give them both the same view transition name.
Here is the HTML of the two pages:
But will the view transition work now?
No, as we mentioned earlier in this post, your view transition names have to be unique on your current page. In this case, on the index page, we have six thumbnail elements ( .index-thumbnail ). Giving all of them the same name thumbnail in the CSS will break the transition. So, what's the solution?
In this simple case, when the thumbnail is clicked, we can use JavaScript to apply the view-transition-name property to the elements only during the transition.
This approach guarantees the presence of only one named view transition pseudo-element each time on the page.
Also, we need to remove the .index-thumbnail class from our selectors in CSS to fix the demo:
You can preview the demo here .
When you click on a .index-thumbnail element, it will get the same name as its counterpart on the detail page, which has the class .detail-thumbnail . This causes the named view transition to work properly.
Does this make sense now? Imagine if all these thumbnails had the same view transition name – how would the browser know which one to morph into the thumbnail on the next page?
You can find the source code of this demo. The script can be found at the end of the index.html file.
The best way to turn off a view transition is to set the view-transition-name property of the element to none .
When we assign a name to elements using this property, they receive view transitions. This even applies to the root element, which is automatically set to root when we activate the View Transitions API on the page.
This is the default style from the user agent, and we can cancel it out by giving it a value of none to disable its view transition like this:
You could come across a few surprising things when using the View Transitions API. I faced an issue where elements didn't scale correctly during a transition. Let's look at an example to understand this better.
Imagine you have a thumbnail that you want to enlarge only in its width on the next page. Here's the demo:
Let's use Chrome DevTools to slow down the animation and see what's happening. In DevTools, press Cmd/Ctrl + Shift + P , search for "animation," and you can debug any ongoing animation on the page. I'll slow down the animation to understand the issue:
Box1 is maintaining its aspect ratio and resizing proportionally, which isn't the desired behavior. We want its height to remain the same while growing in width.
It's important to remember that the element itself isn't morphing into a different element. Instead, it's a snapshot of that element, particularly the ::view-transition-old part in the View Transitions API when the red box (Box 1) appears during the animation. This snapshot is a rasterized image and tends to keep its ratio while resizing.
Now that we understand the snapshot keeps its aspect ratio when we don't want it to, the solution is to tell the snapshot to always have the same height as the real element. We can do this by setting the height to 100%:
Let’s debug the animation again:
Excellent, the problem is solved! However, we encounter the same issue when transitioning from page 2 to page 1.
This happens because we only changed the height of ::view-transition-old(box) . This pseudo-element represents the snapshot of Box1 when moving from page 1 to page 2. But when going from page 2 back to page 1, Box1 is in the new state, not the old one. So, we should target ::view-transition-new(box) to adjust the snapshot of Box1 in the new state (page 1).
This makes the old and new snapshots fit snugly within the Box itself.
Now, our animation behaves as intended:
Astro is one of the first meta frameworks that added support for view transitions . In this section, we will learn how to add view transitions in an Astro project.
We'll work with the demo project created during our practical Astro guide and enhance it with view transitions.
Enabling view transitions in Astro
This step is applicable for Astro versions prior to 3.0. If you're using version 3 or above, view transitions are already part of the stable release, and you can skip this step.
First, ensure that your project is set up and running using Astro. To enable view transitions, you need to activate the experimental feature in your configuration.
First, you can upgrade your Astro project like this:
Open your astro.config.js file and add the following line:
Add <ViewTransitions /> component
To apply view transitions globally to your entire site and create the SPA mode, you'll need to include the <ViewTransitions /> component. You can do this by adding the import statement and the component placement in your common <head> or shared layout component. For instance, in our project, we are going to add this to our BaseLayout.astro file. First, let’s import the ViewTransitions :
Now, we can use the component like this:
By including this component, you enable the default cross-fade transition effect between different pages of your website.
Add individual element transitions
To have control over different elements of the page, Astro gives you a few transition:* directives that you can use in your .astro components.
To provide more specific and visually pleasing transitions, you can assign unique names to different elements that you want to transition between.
For example, in your Astro components, you can add the transition:name directive to elements you want to have transition. This directive adds the view-transition-name CSS property behind the scenes that helps control the transition.
In our project, we would like to have a separate transition for each blog post's title and image. So here is how we do it:
We want to transition the card's thumbnail into the blog image of the article page.
Since the name has to be unique, I will use the postId of each post at the end of each name.
In the index.astro file:
We do the same for the blog image in the BlogLayout.astro file:
This guarantees unique names for our view transitions and here is the result:
We can do the same thing for our titles as well. You can check out the demo here and find the source code on GitHub .
With these named view transitions, enhancing your websites becomes a breeze. But Astro doesn't stop here—you can further tailor animations using the transition:animate directive to override Astro's default animations. Options include morph , slide , and fade .
You can even customize these animations, as shown below:
Maintaining State
Astro goes the extra mile by allowing you to share JavaScript state between routes. By using the transition:persist directive, you can maintain components and HTML elements across page navigations.
For example, the following <video> element will continue playing even as you navigate to another page containing the same video element. This functionality works for both forward and backward navigation.
Make sure that you read Astro’s documentation on View Transitions . They have more features available that we can’t fit into this article. As Astro evolves, keep an eye out for updates and optimizations that further improve this feature.
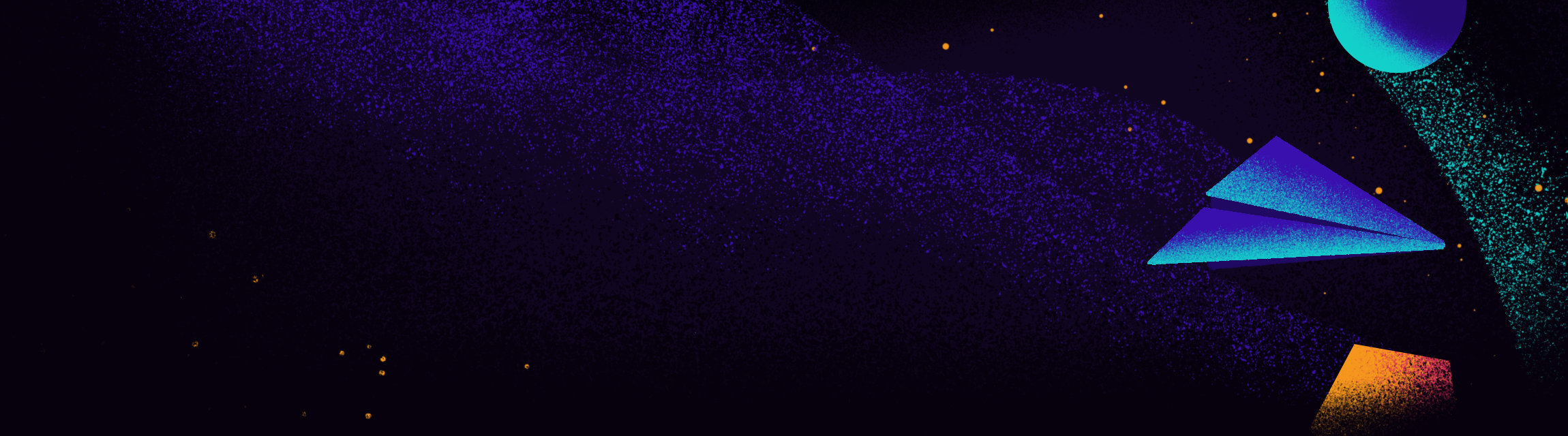
Join Bejamas newsletter!
Get the exclusive modern web dev tutorials and case studies in your mailbox!
In Nuxt.js , support for View Transitions was introduced as an experimental feature in the Nuxt 3.4 release , which is still actively being developed, so anticipate potential future changes.
To enable the View Transitions API in Nuxt, you'll need to make an addition to the nuxt.config.js file:
Once enabled, you get the default cross-fade. You can also apply transitions to specific elements in a manner similar to how you work with CSS. Simply use the style tag within your .vue files like this:
The Nuxt team has provided a demo that you can explore on Stackblitz :
SvelteKit , starting from version 1.24, has made a way to integrate the View Transitions API for view transitions.
To enable view transitions in SvelteKit, you can use a navigation lifecycle function called the onNavigate hook, which fires on every navigation just before the new page is rendered.
Add the following code to your src/routes/+layout.svelte file:
Now, every navigation will trigger a view transition.
You can read more about this on the Svelte blog .
You can check the browser support on caniuse.com . Only Chrome and Edge support the View Transitions API at the time of writing. However, this shouldn't discourage you from considering its use. Progressive enhancement can be a helpful strategy in this situation.
With progressive enhancement, you can add extra features and fancier stuff on top of that basic foundation. This way, users with modern browsers will get to experience the extra cool features, while users with other browsers still get a functional and usable site.
So, even though the View Transitions API might not be supported by all browsers, using the progressive enhancement approach allows you to offer a great experience to everyone while taking advantage of the API's features for those who can enjoy them.
Creating smooth page transitions has always been a goal in web development. The View Transitions API is a big step forward in achieving this. It lets developers add attractive animations to their websites.
If you want to dig deeper into understanding the View Transitions API, then by all means, put some time into reading Jake Archibald’s article on the subject. He is one of the main people behind this API. In this post, I explained what I learned from that article in my own simple words.
Readers also enjoyed
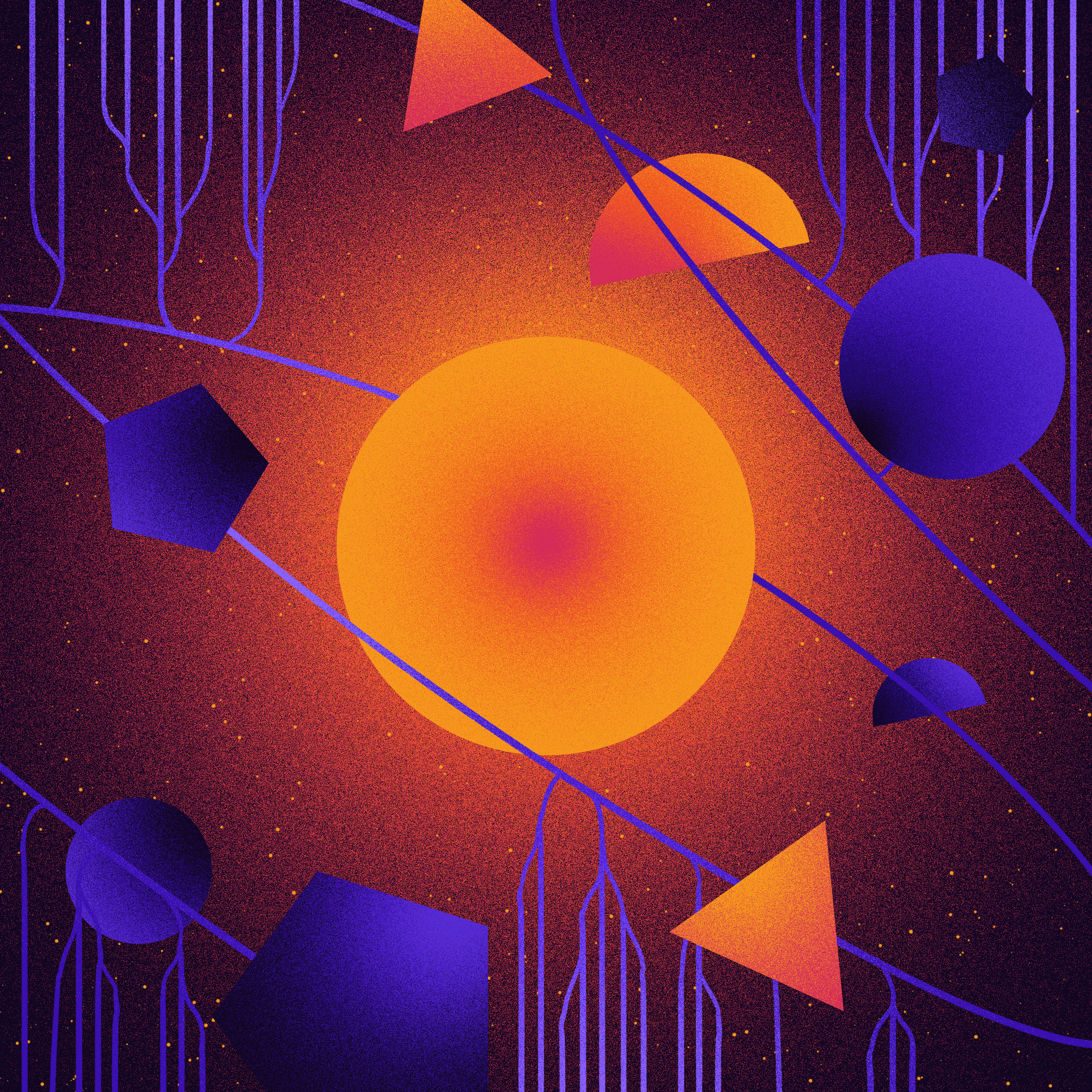
What is Astro JS Framework: A Practical Guide To Building Faster Websites
Learn Astro JS by creating a blog using this modern JavaScript framework and static site generator. Find the benefits of using Astro in web development.
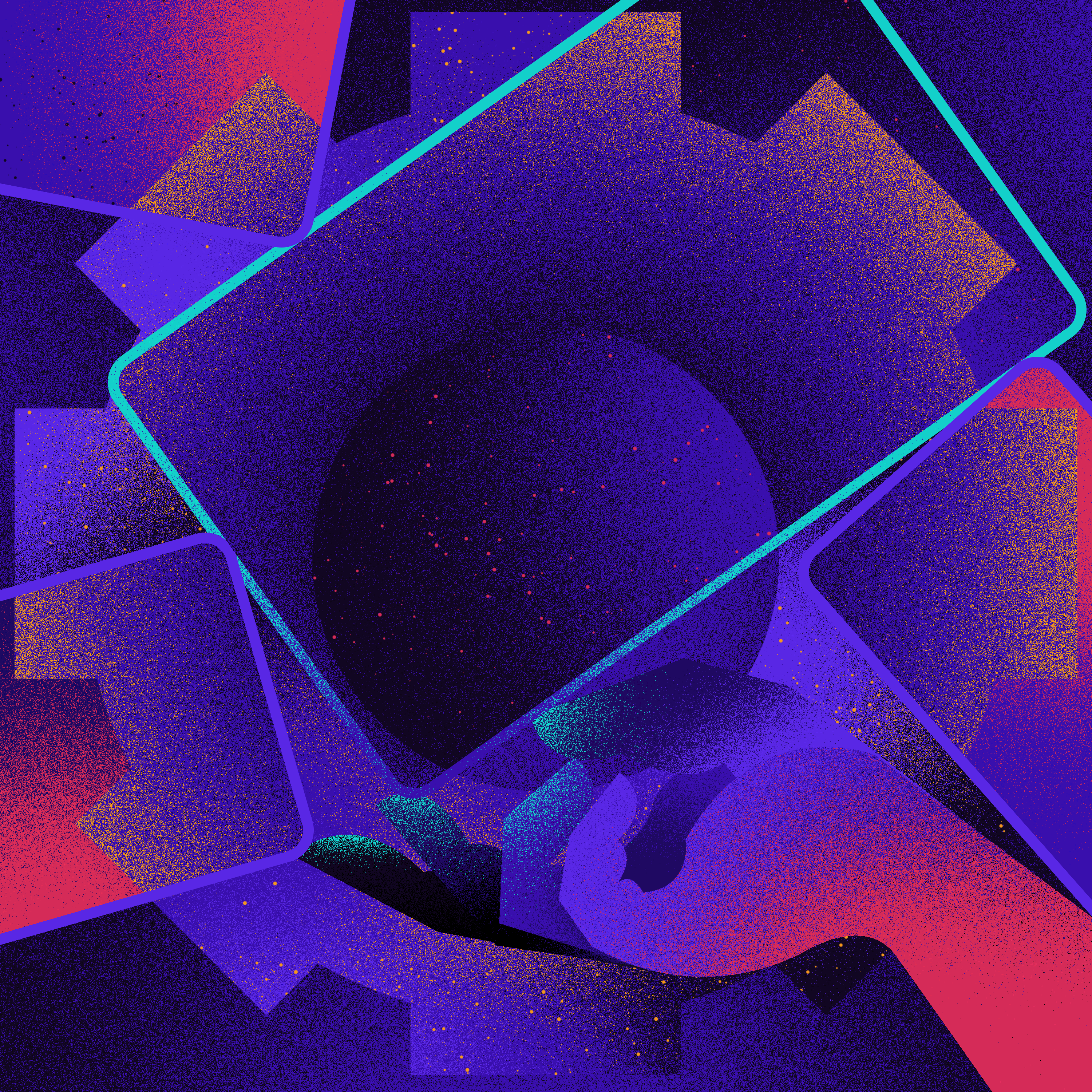

Building a sustainable website for Dodonut using Astro JS
Discover how Bejamas used the Astro framework to create Dodonut's low carbon footprint website. Explore its unique features for sustainable web design and learn about its benefits for your own projects.
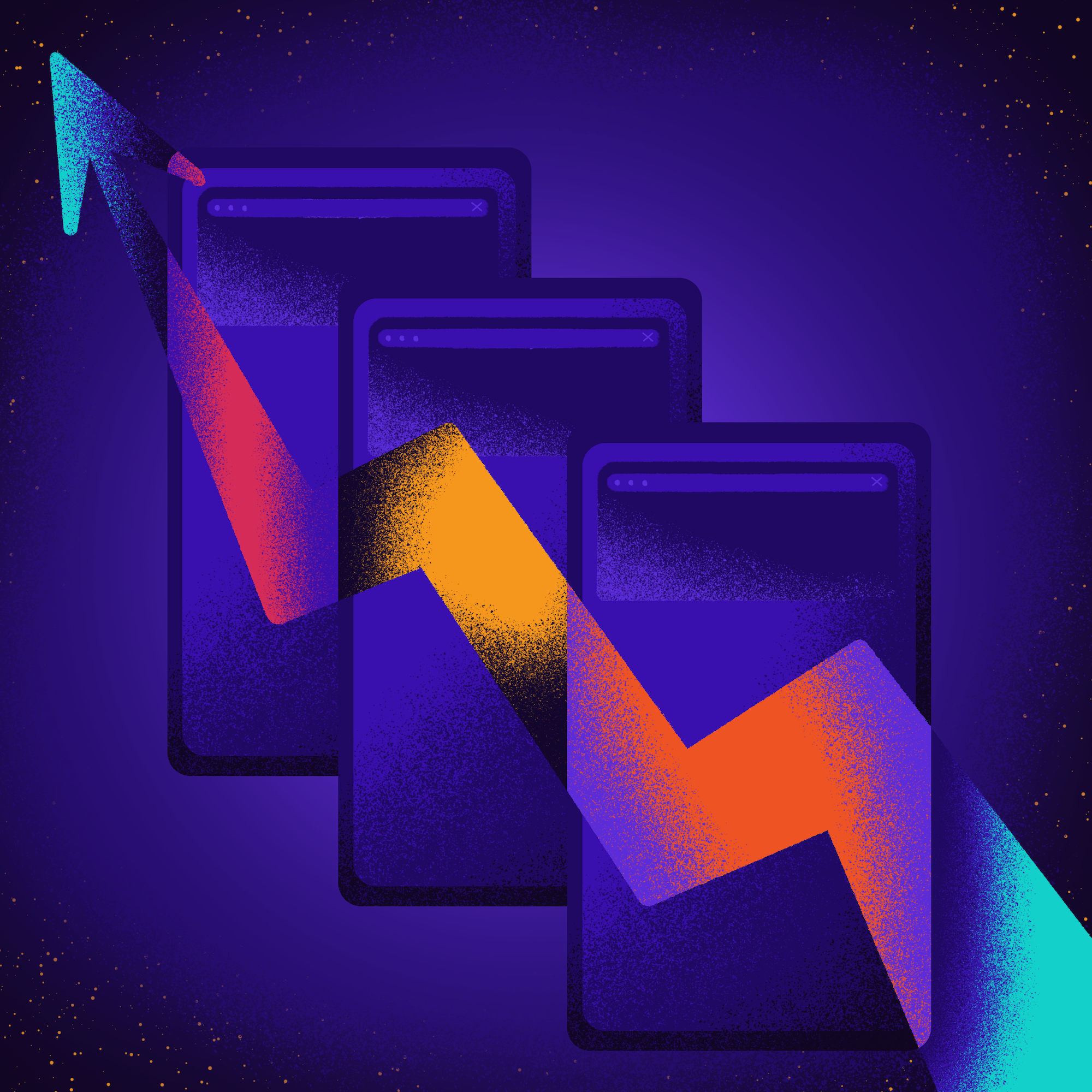
Moving Backlinko to Headless WordPress and Next.js
SEO businesses feel the Jamstack vibes. When Google announced their upcoming Page Experience update, Backlinko realized that they needed to improve their website. We are happy we could help.
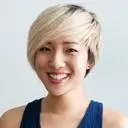
Saxophone House
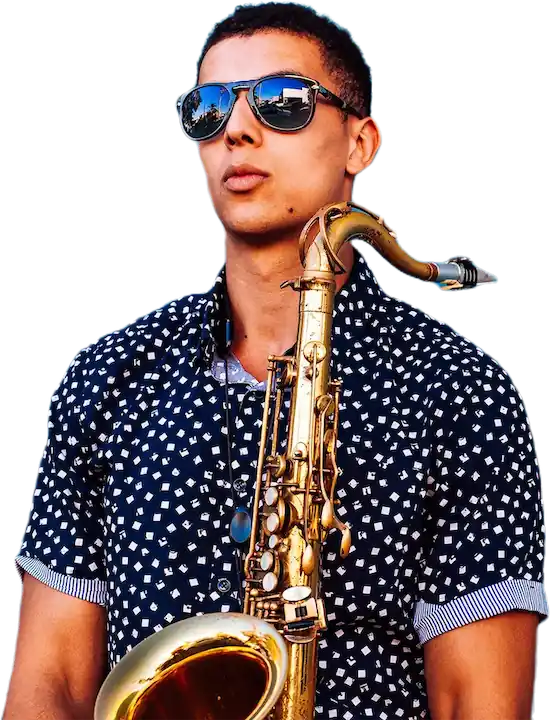
Feel-Good Indie Rock
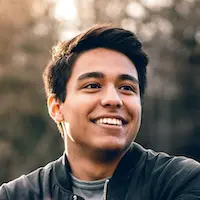
Peaceful Guitar
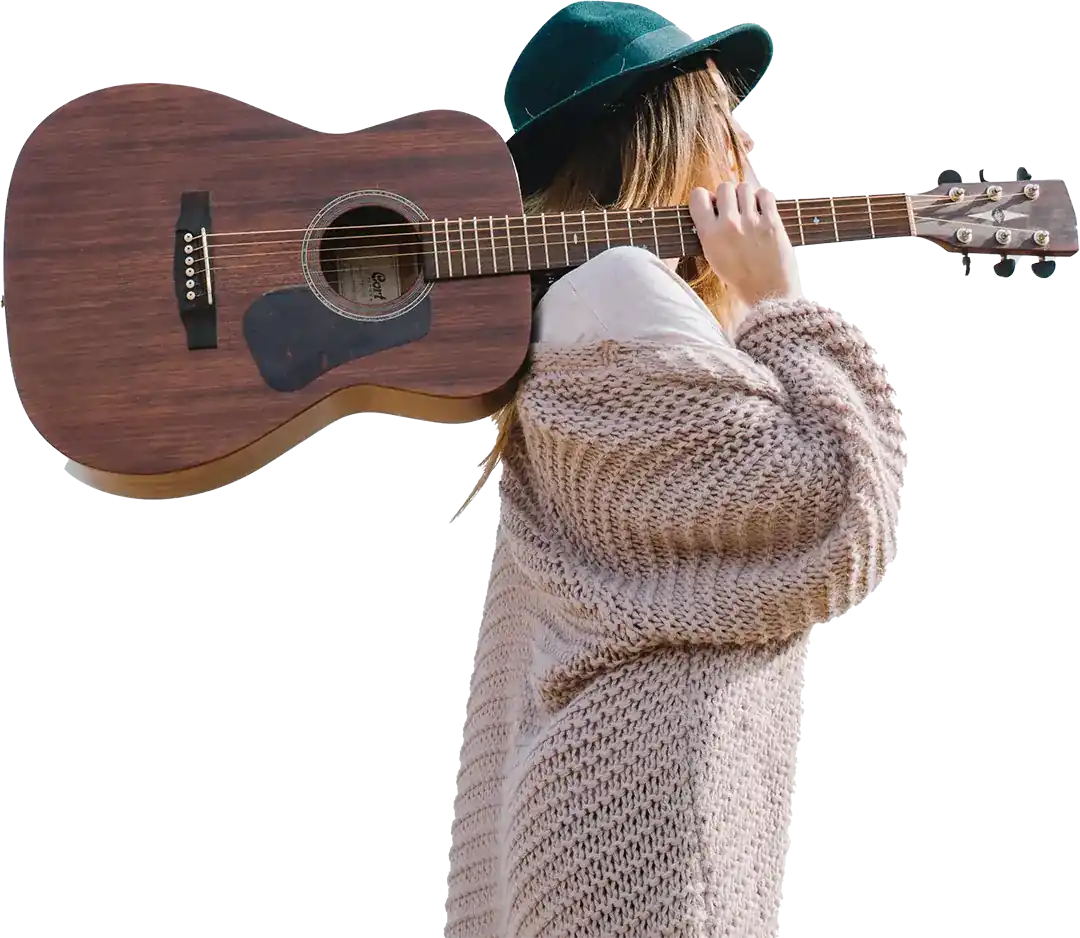
- Adrian Bece
- Jan 2, 2024
The View Transitions API And Delightful UI Animations (Part 2)
- 28 min read
- CSS , API , Animation , UI , Techniques
- Share on Twitter , LinkedIn
About The Author
Adrian Bece is a full-stack web developer with extensive eCommerce experience. He enjoys writing and talking about the latest and greatest technologies in web … More about Adrian ↬
Email Newsletter
Weekly tips on front-end & UX . Trusted by 200,000+ folks.
Last time we met, I introduced you to the View Transitions API . We started with a simple default crossfade transition and applied it to different use cases involving elements on a page transitioning between two states. One of those examples took the basic idea of adding products to a shopping cart on an e-commerce site and creating a visual transition that indicates an item added to the cart.
The View Transitions API is still considered an experimental feature that’s currently supported only in Chrome at the time I’m writing this, but I’m providing that demo below as well as a video if your browser is unable to support the API.
See the Pen [Add to cart animation v2 - completed [forked]](https://codepen.io/smashingmag/pen/GReJGYV) by Adrian Bece .
That was a fun example! The idea that we can effectively take “snapshots” of an element’s before and after states and generate a transition between them using web standards is amazing.
But did you know that we can do even more than transition elements on the page? In fact, we can transition between two entire pages . The same deal applies to single-page applications (SPA): we can transition between two entire views . The result is an experience that feels a lot like an installed mobile app and the page transitions that have been exclusive to them for some time.
That’s exactly what we’re going to cover in the second half of this two-part series. If you want to review the first part , please do! If you’re ready to plow ahead, then come and follow along.
A Quick Review
The browser does a lot of heavy lifting with the View Transitions API, allowing us to create complex state-based UI and page transitions in a more streamlined way. The API takes a screenshot of the “old” page, performs the DOM update, and when the update is complete, the “new” page is captured.
It’s important to point out that what we see during the transition is replaced content in CSS , just the same as other elements, including images, videos, and iframes. That means they are not actually DOM elements, and that’s important because it avoids potential accessibility and usability issues during the transition.
With this little bit of JavaScript, we can call the document.startViewTransition function, and we get the View Transition API’s default animation that performs a crossfading transition between the outgoing and incoming element states.
What we need to do, though, is tell the View Transitions API to pay attention to certain UI elements on the page and watch for their position and dimensions . This is where the CSS view-transition-name property comes in. We apply the property to a single element on the page while the transition function is running; transition names must be unique and applied once per page — think of them like an id attribute in HTML.
That being said, we can apply unique view transition names to multiple elements during the transition. Let’s select one element for the time being — we’ll call it .active-element — and give it a view transition name:
We could do this in JavaScript instead:
When we decide to set the transition name, the element itself becomes what’s called a transition element between the outgoing and incoming state change. Other elements still receive the crossfade animation between outgoing and incoming states.
From here, we can use CSS to customize the animation. Specifically, we get a whole new set of pseudo-elements we can use to target and select certain parts of the transition. Let’s say we have a “card” component that we’ve identified and given a transition name. We can define a set of CSS @keyframes , set it on the two states ( ::view-transition-image-pair(card-active) ), then configure the animation at different levels, such as applying a certain animation timing function, delay, or duration to the entire transition group ( ::view-transition-group(*) ), the “old” page (::view-transition-old(root) ), or the “new” page ( ::view-transition-new(root) ).
Notice how we’re selecting certain UI elements in each pseudo-element, including the entire transition group with the universal selector ( * ), the root of the old and new states, and the card-active we used to name the watched element.
Before we plow ahead, it’s worth one more reminder that we’re working with an experimental web feature. The latest versions of Chrome, Edge, Opera, and Android Browser currently support the API . Safari has taken a positive position on it, and there’s an open ticket for Firefox adoption . We have to wait for these last two browsers to formally support the API before using it in a production environment.
And even though we have Chromium support, that’s got a bit of nuance to it as only Chrome Canary supports multi-page view transitions at the moment behind the view-transition-on-navigation flag. You can paste the following into the Chrome Canary URL bar to access it:
Full View Transitions For Multi-Page Applications
Let’s start with multi-page applications (MPA) . The examples are more straightforward than they are for SPAs, but we can leverage what we learn about MPAs to help understand view transitions in SPAs. Specifically, we are going to build a static site with the Eleventy framework and create different transitions between the site’s different pages.
Again, MPA view transitions are only supported in Chrome Canary view-transition-on-navigation flag. So, if you’re following along, be sure you’re using Chrome Canary with the feature flag enabled. Either way, I’ll include videos of what we’re making to help demonstrate the concepts, like this one we’re about to tackle:
That looks pretty tricky! But we’re starting with the baseline default crossfade animation for this transition and will take things one step at a time to see how everything adds up.
Starting With The Markup
I want to showcase vanilla JavaScript and CSS implementations of the API so that you can apply it to your own tech stack without needing to re-configure a bunch of stuff. Even If you are unfamiliar with Eleventy, don’t worry because I’ll use the compiled HTML and CSS code in the examples so you can follow along.
Let’s check out the HTML for a .card element. Once Eleventy generates HTML from the template with the data from our Markdown files, we get the following HTML markup.
So, what we’re working with is a link with a .card class wrapped around a <figure> that, in turn, contains the <img> in a <picture> that has the <figcaption> as a sibling that also contains its own stuff, including <h2> and <h3> elements. When clicking the .card , we will transition between the current page and the .card ’s corresponding link.
Crossfading Transitions
Implementing crossfade transitions in MPAs is actually a one-line snippet. In fact, it’s a <meta> tag that we can drop right into the document <head> alongside other meta information.
We can still use the document.startViewTransition function to create on-page UI transitions like we did in the examples from the previous article. For crossfade page transitions, we only need to apply this HTML meta tag, and the browser handles the rest for us!
Keep in mind, however, that this is what’s currently only supported in Chrome Canary. The actual implementation might be changed between now and formal adoption. But for now, this is all we need to get the simple crossfade page transitions.
I have to point out how difficult it would be to implement this without the View Transitions API. It’s amazing to see these app-like page transitions between standard HTML documents that run natively in the browser. We’re working directly with the platform!
Transitioning Between Two Pages
We’re going to continue configuring our View Transition with CSS animations. Again, it’s awesome that we can resort to using standard CSS @keyframes rather than some library.
First, let’s check out the project pages and how they are linked together. A user is capable of navigating from the homepage to the item details page and back, as well as navigating between two item details pages.
Those diagrams illustrate (1) the origin page, (2) the destination page, (3) the type of transition, and (4) the transition elements. The following is a closer look at the transition elements, i.e., the elements that receive the transition and are tracked by the API.
So, what we’re working with are two transition elements: a header and a card component . We will configure those together one at a time.
Header Transition Elements
The default crossfade transition between the pages has already been set, so let’s start by registering the header as a transition element by assigning it a view-transition-name . First, let’s take a peek at the HTML:
When the user navigates between the homepage and an item details page, the arrow in the header appears and disappears — depending on which direction we’re moving — while the title moves slightly to the right. We can use display: none to handle the visibility.
We’re actually registering two transition elements within the header: the arrow ( .header__link--dynamic ) and the title ( .header__title ). We use the view-transition-name property on both of them to define the names we want to call those elements in the transition:
Note how we’re wrapping all of this in a CSS @supports query so it is scoped to browsers that actually support the View Transitions API. So far, so good!
Card Transition Element
Turning our attention to the card component, it’s worth recalling that all view transitions on a page must have unique names . So, rather than set the card’s name up front in CSS, let’s instead assign it to the card once the card’s image is clicked to help avoid potential conflicts.
There are different ways to assign a view-transition-name to the clicked card. For example, we can use mouse events. For this demo, however, I’ve decided to use the Navigation API because it’s a good excuse to work with it and put its ability to track back and forward browser navigation to use. Specifically, we can use it to intercept a navigation event and use a query selector on the card image containing the matching target href that has been clicked on to assign a name for the transitioning element.
The item details page is our destination, and we can assign the view-transition-name property to it directly in CSS since it is always going to be a matching image.
We can also customize the animations we’ve just created using standard CSS animation properties. For now, let’s merely play around with the animation’s duration and easing function.
And just like that, we have created a neat page transition! And all we really did was assign a couple of transition elements and adjust their duration and timing functions to get the final result.
Working With More Complex Animations
Let’s move on to additional, more complex animations that run after the page transition has finished. We won’t actually use them just yet, but we are setting them up so that we can use them for transitioning between two product details pages.
Why are we going with the CSS animations , all of a sudden? If you recall from the first article in this two-part series , the page is not interactive while the View Transitions API is running . Although the transition animations look smooth and gorgeous, we want to keep them as short as possible so we don’t make the user wait for too long to interact with the page. We also want to be able to interrupt the animation when the user clicks on a link.
The following CSS defines two sets of animation @keyframes : one for the album to open up its cover, and another for the album itself to rollOut of the sleeve.
Check it out! Our CSS animations are now included in the transitions.
We aren’t quite done yet. We haven’t actually applied the animations. Let’s do that before playing with the animation a little more.
Transitioning Between Two Items Linked To Different Pages
OK, so we’ve already completed an example of a view transition between two pages in an MPA. We did it by connecting the site’s global navigation to any product details page when clicking either on the header link or the card component.
We also just created two CSS animations that we haven’t put to use. That is what we’ll do next to set a view transition when navigating between two product pages. For this one, we will create a transition on the product images by clicking on the left or right arrows on either side of the product to view the previous or next product, respectively.
To do that, let’s start by defining our transition elements and assign transition names to the elements we’re transitioning between the product image ( .product__image--deco ) and the product disc behind the image ( .product__media::before ).
Notice how we had to remove the crossfade animation from the product image’s old ( ::view-transition-old(product-lp) ) and new ( ::view-transition-new(product-lp) ) states. So, for now, at least, the album disc changes instantly the moment it’s positioned back behind the album image.
But doing this messed up the transition between our global header navigation and product details pages. Navigating from the item details page back to the homepage results in the album disc remaining visible until the view transition finishes rather than running when we need it to.
The way we fix this is by removing transitions when returning to a previous state. When we’re working with elaborate page transitions like this one, we have to be mindful of all the different types of navigation paths that can occur and ensure transitions run smoothly regardless of which route a user takes or which direction they navigate.
Just like we can assign view-transition-name attributes when needed, we can also remove them to restore the element’s default crossfade transition. Let’s once again use the Navigation API, this time to intercept the navigation event on the item details page. If the user is navigating back to the homepage, we’ll simply set the view-transition-name of the album disc element to none to prevent conflicts.
Now, all our bases are covered, and we’ve managed to create this seemingly complex page transition with relatively little effort! The crossfade transition between pages works right out of the box with a single meta tag added to the document <head> . All we do from there is set transition names on elements and fiddle with CSS animation properties and @keyframes to make adjustments.
The following demo includes the code snippets that are directly relevant to the View Transitions API and its implementation. If you are curious about the complete codebase or want to play around with the example, feel free to check out the source code in this GitHub repository . Otherwise, a live demo is available below:
- Open Live Demo 1
Full View Transitions For Single-Page Applications
The View Transition API gets a little tricky in single-page applications (SPA) . Once again, we need to rely on the document.startViewTransition function because everything is handled and rendered with JavaScript. Luckily, routing libraries exist, like react-router , and they have already implemented page transitions with the View Transitions API as an opt-in. Other libraries are following suit.
In this next tutorial, we’ll use react-router to create the transitions captured in the following video:
There are a few different types of transitions happening there, and we are going to make all of them. Those include:
- Transition between category pages;
- Transition between a category page and a product details page;
- Transition the product image on a product details page to a larger view.
We’ll begin by setting up react-router before tackling the first transition between category pages.
React Router Setup
Let’s start by setting up our router and main page components. The basic setup is this: we have a homepage that represents one product category, additional pages for other categories, and pages for each individual product.
Let’s configure the router to match that structure. Each route gets a loader function to handle page data.
With this, we have established the routing structure for the app:
- Homepage ( / );
- Category page ( /:category );
- Product details page ( /:category/product/:slug ).
And depending on which route we are on, the app renders a Layout component. That’s all we need as far as setting up the routes that we’ll use to transition between views. Now, we can start working on our first transition: between two category pages.
Transition Between Category Pages
We’ll start by implementing the transition between category pages. The transition performs a crossfade animation between views. The only part of the UI that does not participate in the transition is the bottom border of the category filter menu, which provides a visual indication for the active category filter and moves between the formerly active category filter and the currently active category filter that we will eventually register as a transition element.
Since we’re using react-router, we get its web-based routing solution, react-router-dom , baked right in, giving us access to the DOM bindings — or router components we need to keep the UI in sync with the current route as well as a component for navigational links. That’s also where we gain access to the View Transitions API implementation.
Specifically, we will use the component for navigation links ( Link ) with the unstable_viewTransition prop that tells the react-router to run the View Transitions API when switching page contents.
That is literally all we need to register and run the default crossfading view transition! That’s again because react-router-dom is giving us access to the View Transitions API and does the heavy lifting to abstract the process of setting transitions on elements and views.
Creating The Transition Elements
We only have one UI element that gets its own transition and a name for it, and that’s the visual indicator for the actively selected product category filter in the app’s navigation. While the app transitions between category views, it runs another transition on the active indicator that moves its position from the origin category to the destination category.
I know that I had earlier described that visual indicator as a bottom border, but we’re actually going to establish it as a standard HTML horizontal rule ( <hr> ) element and conditionally render it depending on the current route. So, basically, the <hr> element is fully removed from the DOM when a view transition is triggered, and we re-render it in the DOM under whatever NavLink component represents the current route.
We want this transition only to run if the navigation is visible, so we’ll use the react-intersection-observer helper to check if the element is visible and, if it is, assign it a viewTransitionName in an inline style.
Transitioning Between Two Product Views
So far, we’ve implemented the default crossfade transition between category views and registered the <hr> element we’re using to indicate the current category view as a transition element. Let’s continue by establishing the transition between two product views.
What we want is to register the product view’s main image element as a transition element each time the user navigates from one product to another and for that transition element to actually transition between views. There’s also a case where users can navigate from a product view to a category view that we need to account for by falling back to a crossfade transition in that circumstance.
First, let’s take a look at our Card component used in the category views. Once again, react-router-dom makes our job relatively easy, thanks to the unstable_useViewTransitionState hook. The hook accepts a URL string and returns true if there is an active page transition to the target URL, as well as if the transition is using the View Transitions API.
That’s how we’ll make sure that our active image remains a transition element when navigating between a category view and a product view.
We know which image in the product view is the transition element, so we can apply the viewTransitionName directly to it rather than having to guess:
We’re on a good track but have two issues that we need to tackle before moving on to the final transitions.
One is that the Card component’s image ( .card__image ) contains some CSS that applies a fixed one-to-one aspect ratio and centering for maintaining consistent dimensions no matter what image file is used. Once the user clicks on the Card — the .card-image in a category view — it becomes an .item-image in the product view and should transition into its original state, devoid of those extra styles.
In other words, the transition element is unaware of the CSS that is responsible for those styles and is unable to track it on its own. We need to customize it with CSS pseudo-elements in the same way we did in the previous article of this two-part series .
Jake Archibald shared this simple and effective CSS snippet for handling the aspect ratio changes. We’re going to use it here with some minor adjustments for our specific use case.
Next, we’ll use the unstable_useViewTransitionState to conditionally set a viewTransitionName on the image only when the user navigates from the product view back to the category page for that product.
Let’s keep this example simple and focus solely on how to conditionally toggle the viewTransitionName parameter based on the target URL.
Transitioning Between Image States
It’s time for the third and final transition we identified for this example: transitioning the product image on a product details page to a larger view. It’s actually less of a transition between views than it is transitioning between two states of the image element.
We can actually leverage the same UI transition we created for the image gallery in the last article . That article demonstrated how to transition between two snapshots of an element — its “old” and “new” states — using a grid of images. Click an image, and it transitions to a larger scale.
The only difference here is that we have to adapt the work we did in that example to React for this example. Otherwise, the main concept remains exactly the same as what we did in the last article.
Jake has recommended using React’s flushSync function to make this work. The function forces synchronous and immediate DOM update s inside a given callback. It’s meant to be used sparingly, but it’s okay to use it for running the View Transition API as the target component re-renders.
With this in place, all we really have to do now is toggle class names and view transition names depending on the state we defined in the previous code.
We are applying viewTransitionName directly on the image’s style attribute. We could have used boolean variables to toggle a CSS class and set a view-transition-name in CSS instead. The only reason I went with inline styles is to show both approaches in these examples. You can use whichever approach fits your project!
Let’s round this out by refining styles for the overlay that sits behind the image when it is expanded:
The following demonstrates only the code that is directly relevant to the View Transitions API so that it is easier to inspect and use. If you want access to the full code, feel free to get it in this GitHub repo .
- Open Live Demo 2
We did a lot of work with the View Transitions API in the second half of this brief two-part article series. Together, we implemented full-view transitions in two different contexts, one in a more traditional multi-page application (i.e., website) and another in a single-page application using React.
We started with transitions in a MPA because the process requires fewer dependencies than working with a framework in a SPA. We were able to set the default crossfade transition between two pages — a category page and a product page — and, in the process, we learned how to set view transition names on elements after the transition runs to prevent naming conflicts.
From there, we applied the same concept in a SPA, that is, an application that contains one page but many views. We took a React app for a “Museum of Digital Wonders” and applied transitions between full views, such as navigating between a category view and a product view. We got to see how react-router — and, by extension, react-router-dom — is used to define transitions bound to specific routes. We used it not only to set a crossfade transition between category views and between category and product views but also to set a view transition name on UI elements that also transition in the process.
The View Transitions API is powerful, and I hope you see that after reading this series and following along with the examples we covered together. What used to take a hefty amount of JavaScript is now a somewhat trivial task, and the result is a smoother user experience that irons out the process of moving from one page or view to another.
That said, the View Transitions API’s power and simplicity need the same level of care and consideration for accessibility as any other transition or animation on the web . That includes things like being mindful of user motion preferences and resisting the temptation to put transitions on everything. There’s a fine balance that comes with making accessible interfaces, and motion is certainly included.
- CSS View Transitions Module Level 1 Specification (W3C)
- View Transitions API Explainer (GitHub repo)
- View Transitions API (MDN)
- “ Smooth And Simple Transitions With The View Transitions API ,” Jake Archibald
Smashing Newsletter
Tips on front-end & UX, delivered weekly in your inbox. Just the things you can actually use.
Front-End & UX Workshops, Online
With practical takeaways, live sessions, video recordings and a friendly Q&A.
TypeScript in 50 Lessons
Everything TypeScript, with code walkthroughs and examples. And other printed books.
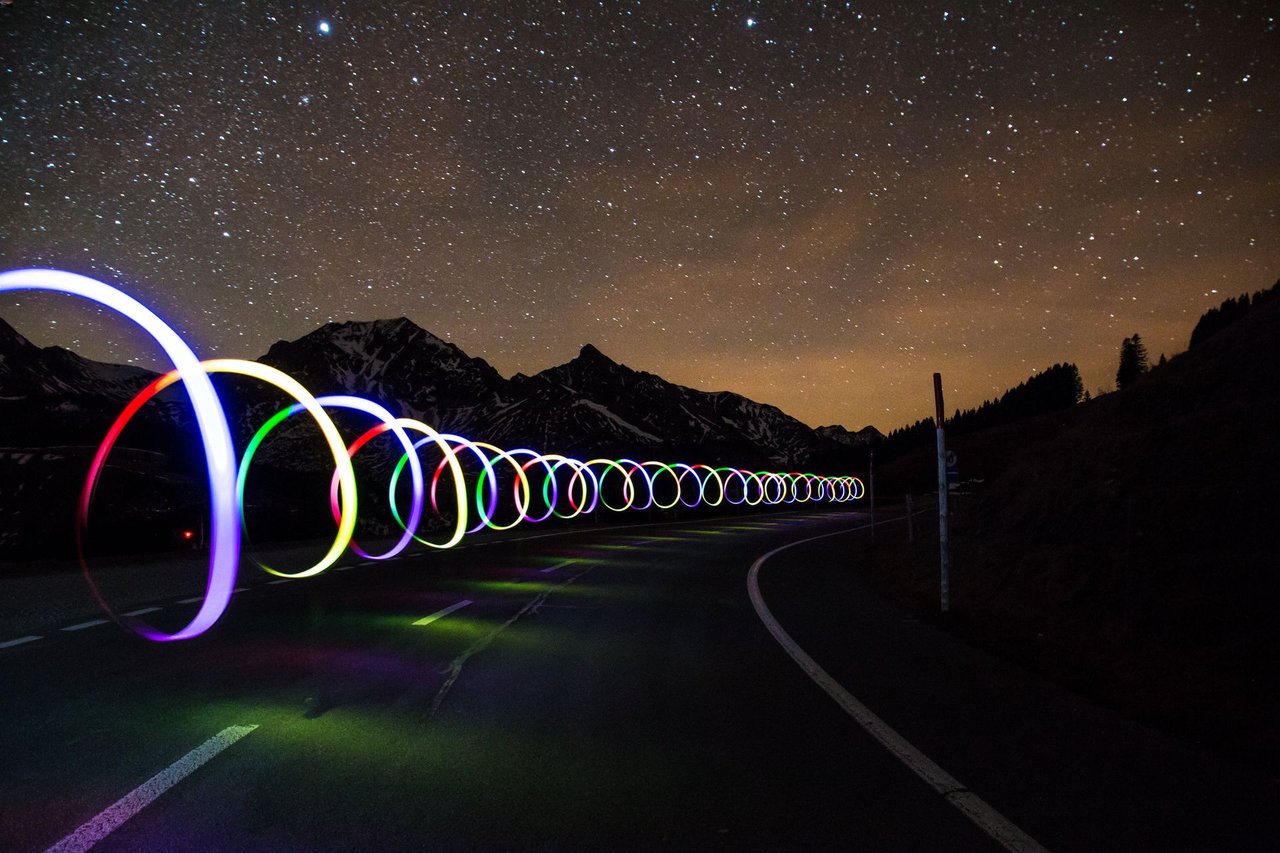
The View Transition API: A New Way to Animate Page Transitions
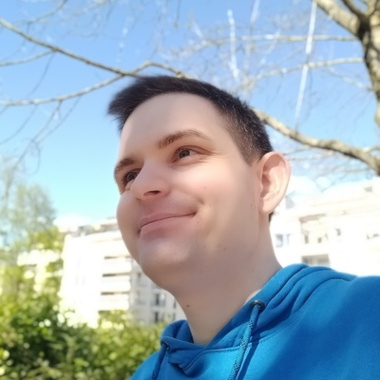
The web is going forward and pages are becoming more and more dynamic. We can see a lot of animations and transitions on websites. It's a good thing because it helps to have a better visual experience. But it's not always easy to implement and maintain. That's why the View Transition API is here to help us. It's an experimental API for view transition that is active on Chromium-based browsers. It allows us to have better control of the transition between two pages.
What Is a View Transition?
A view transition is a visual effect that smoothly changes from one page to another. The browser identifies differences between pages and creates animations, like fading or more complex effects.
View transitions are common in mobile applications. The View Transition API aims to bring this experience to the web.
The following demo shows a security test with 3 questions. Each question is a page. When users answer a question, the page will transition to the next question. Note that the transitions aren't designed to be beautiful, but to show the differences between pages!
View Transitions Basics
To use view transitions, you need to put the page rendering logic in a function and call this function with document.startViewTransition .
The browser will compare the DOM before and after the transition, and animate the differences.
Elements present on both the old and new DOM will move from their original to their new positions. Elements only on the new DOM will appear with a fade-in effect. Elements only on the old DOM will disappear with a fade-out effect.
The following demo shows a simple transition between full-page images using a fade-in/fade-out transition ( source code ).
Customizing Transitions
The View Transition API allows us to customize the transition by using CSS. Transitions are just CSS animations.
Browsers supporting View transitions introduce two new CSS selectors : ::view-transition-old and ::view-transition-new . As their name suggests, these pseudo-elements represent the elements that are only on the old DOM or only on the new DOM.
For instance, to animate the whole page, change the animations on the root transition name:
Both animations will be played at the same time.
Check the Demo and the source code .
Animating An Element Present On Both Pages
In the previous example, you may have noticed the root name in ::view-transition-old(root) . This represents the animation to play for the whole page.
We can create different animations for different elements by giving them a unique transition name . In the following example, we will make a custom animation for an image description.
The example has some more CSS to customize the header, but here is the result of the same animation.
Demo and the source code .
Moving Elements Between Pages
In this example, we will move one element from the old page to the new page. The item in the list will become the main object of the page.
This works by using the same transition name on both pages. The browser will try to match the elements with the same transition name and animate them.
See the Demo and source code .
And that's it. With the presented elements, you can already do beautiful view transitions. There are many more details to discover. For example, startViewTransition does return an object. The object contains the state of the animation and promises of completion.
For more advanced transitions, I invite you to check the chrome developer page on view transitions and the MDN page on view transitions .
View Transitions In React with React Router or Remix
View Transitions are supported by React Router since v6.17.0 and Remix since v2.1.0 , behind an experimental flag.
As React Router and Remix are using the same API, we will use React Router for the following example, but it's the same for Remix.
React Router added a prop called unstable_viewTransition in the <Link> and <NavLink> components. When using <NavLink> , this property appends the class transitioning to the link when the transition is starting. It allows to customize the link during the transition, and to have custom transitions depending on the link.
The following example shows how to use unstable_viewTransition with <NavLink> and CSS.
You can also use a new hook called unstable_useViewTransitionState(addressTo) that returns true if the navigation is in progress to the given address.
Here is an example of an image link that expands when the user clicks on it.
But you don't necessarily need to use the hook, you can just use the <Link> component as usual and use basic CSS to customize the transition. It's useful to deduplicate the code if you want to animate an item in a list.
I made some riddles with React Router and the View Transition API. You can check the source code on Github and the demo on Github Pages .
The View Transition API is a great way to make beautiful transitions between pages. It's easy to use and it's already supported by Chromium-based browsers. It's a good way to make your website more dynamic and beautiful.
But it's still an experimental API, so it's not recommended to use it in production. Also, one of the major drawbacks is that the page is not interactive while the animation is playing. So it's not recommended to use it for a long animation, but it's perfect for a short animation.
You can already use it in your side projects or for fun.
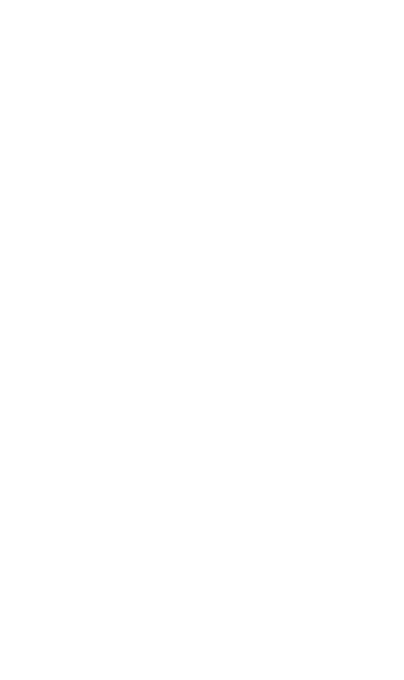
Native Page Transitions With SvelteKit Using The View Transitions API
Published Sep 8, 2023
Table of Contents
What is the view transitions api, animating page transitions, animating state transitions, respect the motion preference for users.
The View Transitions API is a new browser API that lets you easily create animated transitions between different states in your app.
You can find the source code on GitHub .
In the past you had to reach for the FLIP animation technique if you wanted to do impossible layout animations but it wasn’t easy until now.
The View Transitions API makes it easy to create state and page transitions for single-page (SPA) and traditional multi-page applications (MPA). It works by creating a snapshot of the DOM before and after the change and does a cross-fade by default, but it can be customized with CSS transitions.
You can read Smooth and simple transitions with the View Transitions API which includes a lot of examples to learn everything about the View Transitions API. At the time of writing the View Transitions API is only supported in Chromium based browsers, but Safari and Firefox are working on implementing it.
That being said Chrome has the majority share of the browser market at over 60%. If a browser doesn’t support the View Transitions API your site is going to work as normal.
Using the View Transitions API is simple.
This is fine for state transitions but to animate page transitions we have to know when the page changed.
To know when the page changed we can use the onNavigate lifecycle function in SvelteKit.
If the browser doesn’t support the View Transitions API we don’t do anything.
If the browser supports the View Transitions API we return a promise to wait for the page to navigate and load the data. After that is done we resolve the promise and do the transition.
🐿️ If you’re using TypeScript you’re going to get an error because the View Transitions API types don’t exist yet in which case you can add a @ts-expect-error or @ts-ignore line comment to ignore the error.
To control the animation there’s a new ::view-transition pseudo-element that has the selectors for the old and new transition.
You can target the pseudo-elements with CSS to customize the transition.
You can use the animations tab in your developer tools to inspect view transitions.
You can make the page fly in and out of view but also do other fun transitions like the Star Wars wipe effect using CSS blend modes , or the Batman transition .
If you don’t want to use page transitions you can set view-transition-name to none on :root .
The view-transition-name value can be anything including none .
If you don’t want the header to be included in the page transition you can separate it from the rest of the page.
This is how simple it is to animate the active page marker.
You can also animate different DOM elements between page transitions like the planet title and image.
You need to give the view-transition-name a unique name to know what changed which is why we set the name using CSS variables.
The same goes for the new elements your old elements are going to transition to.
The View Transitions API is not only useful for animating page transitions but also animating state transitions.
Let’s use the View Transitions API to animate the state change when a user does a reservation for a flight to some planet.
That’s how simple animating state transitions is, and it only required a couple of lines of code.
SvelteKit gives you complete control over the View Transitions API but in the future there might be an abstraction you can like the sveltekit-view-transition project from @PaoloRicciuti .
With great power comes great responsibility.
Don’t forget to respect the motion preference for users but keep in mind that prefers-reduced-motion doesn’t mean no motion.
That’s it! 😄
If you want to support the content you're reading or watching on YouTube consider becoming a patreon starting low as 1$ per month .
Every post is a Markdown file so contributing is simple as following the link below and pressing the pencil icon inside GitHub to edit it.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
View Transitions API Level 1 #48
khushalsagar commented Aug 22, 2022 • edited by nt1m
- 👍 71 reactions
- 👀 7 reactions
khushalsagar commented Sep 8, 2022
Sorry, something went wrong.
khushalsagar commented Sep 13, 2022
fwebdev commented Mar 14, 2023
astearns commented Mar 14, 2023
gugadev commented Jul 27, 2023
- 👀 5 reactions
smfr commented Aug 15, 2023 • edited
- 🎉 113 reactions
- ❤️ 35 reactions
rik commented Oct 26, 2023
- 👍 8 reactions
annevk commented Oct 31, 2023
- 👍 2 reactions
bokand commented Jan 11, 2024 • edited
nt1m commented Jan 11, 2024
- 👍 3 reactions
No branches or pull requests
Getting started with View Transitions on multi-page apps
May 18, 2023
Spurred by last week’s ShopTalk I rolled out View Transitions here on my static Jekyll site. I hadn’t realized View Transitions for multi-page apps (MPAs) and static sites are ready for testing behind a flag in Chrome 113+. View Transitions for MPAs are a feature that’s high on my CSS wishlist, so I got to it. It took less than an hour to do, requires zero JavaScript, and two lines of CSS. I’m pleased with the results.
⚠️ At the time of writing this only work well in Chrome Canary 115+. I cannot fix this for you in other browsers. For browsers that don’t have multi-page View Transitions turned on by default ( literally all of them ) your pages will load like normal pages. This technique is a ✨ wonderful progressive enhancement.
Let’s get started adding View Transitions to our site…
1. Enable flags in Chrome Canary
To get started with View Transitions, enable them in Chrome Canary.
⚠️ These flags also exist in Chrome Stable and Arc (but not Edge?) but the page will randomly lock up, so stick with Canary or until Chrome Stable hits v115 around Jul 12, 2023. It’s not announced when/if they will unflag this in future releases.
Now we get to the fun part…
2. Include the view-transition meta tag
Add the following to the global <head> of your layout template.
Congrats, you now have page transitions! This one-liner applies a crossfade effect across your entire site. Your website is now a PowerPoint. It’s unclear to me if the <meta> tag will be the final solution to enable this functionality, but I’m happy to see it works.
3. Add individual element transitions
You’re probably asking yourself two questions:
- How do I create an effect more exciting than a crossfade?
- How do I transition specific elements?
The answer to that is: named view transitions in CSS. Give the element you want to transition from (on page1.html ) and element you want to transition to (on page2.html ) the same unique view-transition-name . Let’s use a Bootstrap-style “ Jumbotron ” as an example.
On product.html find the target element you want your element to morph into and give it the same view-transition-name
Voilà! This gives you a “morph” effect between the two pages. If you hate the inline styles, this can be done in a CSS file as well.
And if you want to be a real champ, disable the morph effect for people who get motion sickness . 🏆
Before jumping into making our own custom transitions, let’s pause for a minute and look at how this all works and learn about any limitations in the underlying technology…
How does this all work?
Behind the scenes the browser is rasterizing (read: making and image of ) the before and after states of the DOM elements you’re transitioning. The browser figures out the differences between those two snapshots and tweens between them similar to Apple Keynote’s “Magic Morph” feature, the liquid metal T-1000 from Terminator 2: Judgement Day , or the 1980s cartoon series Turbo Teen .
If those references are lost on you, how about the popular kids book series Animorphs ?
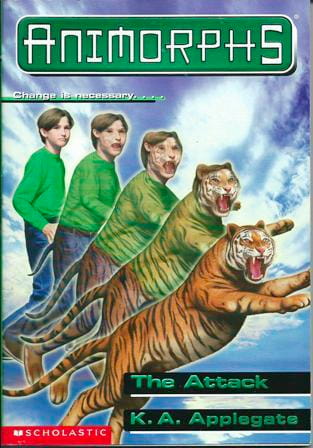
This is exactly how it works and no I won’t be taking any further questions. Anecdotally, it appears to negotiate the X,Y position and the height/width of the before and after states. It will then hot swap your DOM for rasterized images (like transitions), resize transform the height/width, translate the position, and crossfade animation between the old and new states. Basic but convincing.
Unique limitations for lists of many items
Right now MPA view-transitions need to be unique! If you have two elements on the same page with the same transition name (e.g. view-transition-name: post ) the browser gets confused and will bail on the view transition, falling back to cross-fade.
Thankfully there’s a workaround if you’re trying to animate from a list-view to a details-view and back by adding a unique view-transition-name in your template. This example is a list of posts:
This view transition will have the name of post-123 in your rendered HTML. You then add the same “slug” to your post template to morph the link in to the article header.
Now you’ve hacked the system and are able to transition as you’d expect. One side effect to be aware of is that this creates a lot of pseudo-elements in your Web Inspector…
I imagine this is fairly moot, but could also be similar to a “too many DOM nodes” performance and debugging issue. You do what you want, but I’m probably going to be conservative about this and avoid applying it on gigantic lists. I’m hoping a better, less manual/explicit API for list-to-detail transitioning comes along, but for now this works.
4. Customize your view transitions
What if the “morph” transition isn’t what you want? You can control your named view transition’s old (outgoing) state and the new (incoming) state using the ::view-transition-old() and ::view-transition-new() pseudo elements.
It’s a little duplicative, but nice to have control over the incoming and outgoing content. Small subtle tweaks like making the old content fade out a little faster than the new content comes in can make your app feel uniquely yours.
I mostly use the default morph transition, but ran into a problem on my About section subnav where I didn’t like how the morph transition was scaling my text due to a difference in content container sizes between pages. Adding width: fit-content to the transition worked wonders and I got a quick horizontal resize effect I’ve wanted for a long time.
Sky’s the limit on how far you want to go with this. Get ready for egregious animation wipes coming to a website near you.
Have fun, progressively
I think the most telling predictor for the success of the multi-page View Transitions API – compared to all other proposals and solutions that have come before it – is that I actually implemented this one. Despite animations being my bread and butter for many years, I couldn’t be arsed to even try any of the previous generation of tools.
The ability to easily – with a <meta> tag and a handful of CSS properties – create quality 60 FPS page transitions is a game changer and I look forward to what more creative people will do with this technology. My head is already spinning with ideas. The best part about MPA View Transitions, is that under the hood it’s just normal “dumb” page loads and we’re progressively enhancing our way into smart, sleek transitions all with zero JavaScript required.
I sure hope other browsers hop on board. 👀
View Transitions API
Experimental: This is an experimental technology Check the Browser compatibility table carefully before using this in production.
The View Transitions API provides a mechanism for easily creating animated transitions between different DOM states while also updating the DOM contents in a single step.
Concepts and usage
View transitions are a popular design choice for reducing users' cognitive load, helping them stay in context, and reducing perceived loading latency as they move between states or views of an application.
However, creating view transitions on the web has historically been difficult. Transitions between states in single-page apps (SPAs) tend to involve writing significant CSS and JavaScript to:
- Handle the loading and positioning of the old and new content.
- Animate the old and new states to create the transition.
- Stop accidental user interactions with the old content from causing problems.
- Remove the old content once the transition is complete.
Accessibility issues like loss of reading position, focus confusion, and strange live region announcement behavior can also result from having the new and old content both present in the DOM at once. In addition, cross-document view transitions (i.e. across different pages in regular, non-SPA websites) are impossible.
The View Transitions API provides a much easier way of handling the required DOM changes and transition animations.
Note: The View Transitions API doesn't currently enable cross-document view transitions, but this is planned for a future level of the spec and is actively being worked on.
Creating a basic view transition
As an example, an SPA may include functionality to fetch new content and update the DOM in response to an event of some kind, such as a navigation link being clicked or an update being pushed from the server. In our Basic View Transitions demo we've simplified this to a displayNewImage() function that shows a new full-size image based on the thumbnail that was clicked. We've encapsulated this inside an updateView() function that only calls the View Transition API if the browser supports it:
This code is enough to handle the transition between displayed images. Supporting browsers will show the change from old to new images and captions as a smooth cross-fade (the default view transition). It will still work in non-supporting browsers but without the nice animation.
It is also worth mentioning that startViewTransition() returns a ViewTransition instance, which includes several promises, allowing you to run code in response to different parts of the view transition process being reached.
The view transition process
Let's walk through how this works:
- When document.startViewTransition() is called, the API takes a screenshot of the current page.
- Next, the callback passed to startViewTransition() is invoked, in this case displayNewImage , which causes the DOM to change. When the callback has run successfully, the ViewTransition.updateCallbackDone promise fulfills, allowing you to respond to the DOM updating.
- The API captures the new state of the page as a live representation.
- ::view-transition is the root of view transitions overlay, which contains all view transitions and sits over the top of all other page content.
- ::view-transition-old is the screenshot of the old page view, and ::view-transition-new is the live representation of the new page view. Both of these render as replaced content, in the same manner as an <img> or <video> , meaning that they can be styled with handy properties like object-fit and object-position .
- The old page view animates from opacity 1 to 0, while the new view animates from opacity 0 to 1, which is what creates the default cross-fade.
- When the transition animation has reached its end state, the ViewTransition.finished promise fulfills, allowing you to respond.
Different transitions for different elements
At the moment, all of the different elements that change when the DOM updates are transitioned using the same animation. If you want different elements to animate differently from the default "root" animation, you can separate them out using the view-transition-name property. For example:
We've given the <figcaption> element a view-transition-name of figure-caption to separate it from the rest of the page in terms of view transitions.
With this CSS applied, the pseudo-element tree will now look like this:
The existence of the second set of pseudo-elements allows separate view transition styling to be applied just to the <figcaption> . The different old and new page view captures are handled completely separate from one another.
The value of view-transition-name can be anything you want except for none — the none value specifically means that the element will not participate in a view transition.
Note: view-transition-name must be unique. If two rendered elements have the same view-transition-name at the same time, ViewTransition.ready will reject and the transition will be skipped.
Customizing your animations
The View Transitions pseudo-elements have default CSS Animations applied (which are detailed in their reference pages ).
Notably, transitions for height , width , position , and transform do not use the smooth cross-fade animation. Instead, height and width transitions apply a smooth scaling animation. Meanwhile, position and transform transitions apply smooth movement animations to the element.
You can modify the default animation in any way you want using regular CSS.
For example, to change the speed of it:
Let's look at something more interesting — a custom animation for the <figcaption> :
Here we've created a custom CSS animation and applied it to the ::view-transition-old(figure-caption) and ::view-transition-new(figure-caption) pseudo-elements. We've also added a number of other styles to both to keep them in the same place and stop the default styling from interfering with our custom animations.
Note that we also discovered another transition option that is simpler and produced a nicer result than the above. Our final <figcaption> view transition ended up looking like this:
This works because, by default, ::view-transition-group transitions width and height between the old and new views. We just needed to set a fixed height on both states to make it work.
Note: Smooth and simple transitions with the View Transitions API contains several other customization examples.
Controlling animations with JavaScript
The document.startViewTransition() method returns a ViewTransition object instance, which contains several promise members allowing you to run JavaScript in response to different states of the transition being reached. For example, ViewTransition.ready fulfills once the pseudo-element tree is created and the animation is about to start, whereas ViewTransition.finished fulfills once the animation is finished, and the new page view is visible and interactive to the user.
For example, the following JavaScript could be used to create a circular reveal view transition emanating from the position of the user's cursor on click, with animation provided by the Web Animations API .
This animation also requires the following CSS, to turn off the default CSS animation and stop the old and new view states from blending in any way (the new state "wipes" right over the top of the old state, rather than transitioning in):
Represents a view transition, and provides functionality to react to the transition reaching different states (e.g. ready to run the animation, or animation finished) or skip the transition altogether.
Extensions to other interfaces
Starts a new view transition and returns a ViewTransition object to represent it.
CSS additions
Provides the selected element with a separate identifying name and causes it to participate in a separate view transition from the root view transition — or no view transition if the none value is specified.
Pseudo-elements
The root of the view transitions overlay, which contains all view transitions and sits over the top of all other page content.
The root of a single view transition.
The container for a view transition's old and new views — before and after the transition.
A static screenshot of the old view, before the transition.
A live representation of the new view, after the transition.
- Basic View Transitions demo : A basic image gallery demo with separate transitions between old and new images, and old and new captions.
- HTTP 203 playlist : A more sophisticated video player demo app that features a number of different view transitions, many of which are explained in Smooth and simple transitions with the View Transitions API .
Specifications
Browser compatibility.
- Smooth and simple transitions with the View Transitions API
- View Transitions API: Creating Smooth Page Transitions
© 2005–2023 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/API/View_Transitions_API

- New comments
- Military Photos
- Russian Military
- Anti-Aircraft
- SA-21/S-400 Triumf
96L6E Radar, S-400
- Oct 18, 2010
Media information
Share this media.
- This site uses cookies to help personalise content, tailor your experience and to keep you logged in if you register. By continuing to use this site, you are consenting to our use of cookies. Accept Learn more…
- Client log in
Metallurgicheskii Zavod Electrostal AO (Russia)
In 1993 "Elektrostal" was transformed into an open joint stock company. The factory occupies a leading position among the manufacturers of high quality steel. The plant is a producer of high-temperature nickel alloys in a wide variety. It has a unique set of metallurgical equipment: open induction and arc furnaces, furnace steel processing unit, vacuum induction, vacuum- arc furnaces and others. The factory has implemented and certified quality management system ISO 9000, received international certificates for all products. Elektrostal today is a major supplier in Russia starting blanks for the production of blades, discs and rolls for gas turbine engines. Among them are companies in the aerospace industry, defense plants, and energy complex, automotive, mechanical engineering and instrument-making plants.
Headquarters Ulitsa Zheleznodorozhnaya, 1 Elektrostal; Moscow Oblast; Postal Code: 144002
Contact Details: Purchase the Metallurgicheskii Zavod Electrostal AO report to view the information.
Website: http://elsteel.ru
EMIS company profiles are part of a larger information service which combines company, industry and country data and analysis for over 145 emerging markets.
To view more information, Request a demonstration of the EMIS service

IMAGES
VIDEO
COMMENTS
The view transition process. Let's walk through how this works: When document.startViewTransition() is called, the API takes a screenshot of the current page. Next, the callback passed to startViewTransition() is invoked, in this case displayNewImage, which causes the DOM to change. When the callback has run successfully, the ViewTransition ...
View Transitions API (single-document) Provides a mechanism for easily creating animated transitions between different DOM states, while also updating the DOM contents in a single step. This API is specific to single-document transitions, support for same-origin cross-document transitions is being planned. All major browser engines are working ...
The View Transition API is designed to 'wrap' a DOM change and create a transition for it. However, the transition should be treated as an enhancement, as in, your app shouldn't enter an 'error' state if the DOM change succeeds, but the transition fails. Ideally the transition shouldn't fail, but if it does, it shouldn't break the rest of the ...
At the time of writing, the View Transitions API is only available in Chrome v104+ and Canary. To activate it, first, navigate to chrome://flags/. Then, enable the Experimental Web Platform features and viewTransition API for navigations. How the View Transitions API works. The magic of how the View Transitions API works happens behind the scenes.
View Transitions API (single-document) - WD. Limited availability across major browsers. Provides a mechanism for easily creating animated transitions between different DOM states, while also updating the DOM contents in a single step. This API is specific to single-document transitions, support for same-origin cross-document transitions is ...
The View Transitions API is a web-based interface that allows developers to create smooth, seamless transitions between different views or states of a web application. It works by providing a set ...
The View Transitions API is a new — but game-changing — feature that allows us to do the types of reactive state-based UI and page transitions that have traditionally been exclusive to JavaScript frameworks. In the first part of this mini two-part series, Adrian Bece thoroughly explains why we need the API and demonstrates its basic usage.
Overview. View Transitions is a proposal for a new web API that allows a simple set of transition animations in both Single-Page Applications (SPAs) and Multi-Page Applications (MPAs). The inspiration for this feature are transitions similar to the ones listed in the Material Design Principles. The intent is to support transitions similar to ...
The View Transitions API brings in a new tool called the view-transition-name property. This CSS property lets you assign the same special name to both of those elements. This name is what helps you create your named view transition. This works for both SPA and MPA applications.
Interactive transitions. An object that drives an interactive animation between one view controller and another. A set of methods that enable an object (such as a navigation controller) to drive a view controller transition. An interface for modifying an animation while it's running.
Astro + View Transitions Demo. Playlists. Annabelle Lucero March 2023 Saxophone House. 9,838-5h 22m. Jessica Houston ... 6h 40m. This site is a demo of how to persist animating elements across navigation events using the View Transitions API and the Navigation API . Both APIs are available in Chrome 111+. Made with ☕️ by ...
The View Transitions API is a new — but game-changing — feature that allows us to do the types of reactive state-based UI and page transitions that have traditionally been exclusive to JavaScript frameworks. In the second half of this mini two-part series, Adrian Bece expands on the demos from the first article to demonstrate how the View Transitions API can be used to transition not just ...
The View Transition API allows us to customize the transition by using CSS. Transitions are just CSS animations. Browsers supporting View transitions introduce two new CSS selectors: ::view-transition-old and ::view-transition-new. As their name suggests, these pseudo-elements represent the elements that are only on the old DOM or only on the ...
At the time of writing the View Transitions API is only supported in Chromium based browsers, but Safari and Firefox are working on implementing it. That being said Chrome has the majority share of the browser market at over 60%. If a browser doesn't support the View Transitions API your site is going to work as normal.
Maybe a bigger question: should we file a new position request for cross-document view transitions as a whole (i.e. view-transitions API level 2) or is this position sufficient (FWIW Mozilla's reusing the same position for both but thought I'd ask) @nt1m @smfr. All reactions.
The View Transitions API is a new feature that simplifies the process of creating animated transitions between different states or views in web applications. This API allows developers to update the web page's content while also animating the changes in a single step. You can also visit the official demo site, Chrome/Edge 111+ only.
1. Enable flags in Chrome Canary. To get started with View Transitions, enable them in Chrome Canary. chrome://flags#view-transition. chrome://flags#view-transition-on-navigation. ⚠️ These flags also exist in Chrome Stable and Arc (but not Edge?) but the page will randomly lock up, so stick with Canary or until Chrome Stable hits v115 ...
The View Transitions API provides a mechanism for easily creating animated transitions between different DOM states while also updating the DOM contents in a single step. ... Safari WebView Android Chrome Android Firefox for Android Opera Android Safari on IOS Samsung Internet; View_Transitions_API: 111: 111: No: No: 97: No: 111: 111: No: No ...
View count 3,734 Comment count 0 Rating 0.00 star(s) 0 ratings Share this media. Facebook Twitter Reddit Pinterest Tumblr WhatsApp Email Link. Copy image link. Copy image BB code. Copy URL BB code with thumbnail. Copy GALLERY BB code. Home. Military Photos. Russian Military. Army. Anti-Aircraft. SA-21/S-400 Triumf. Terms and rules;
Get directions to Yuzhny prospekt, 6к1 and view details like the building's postal code, description, photos, and reviews on each business in the building. Yuzhny prospekt, 6к1 ... Maps API. Personal. Saved places and transport. My maps. Settings. For business. My profile. Add your organization.
View on OpenStreetMap; Latitude. 55.7904° or 55° 47' 25" north. Longitude. 38.4406° or 38° 26' 26" east. Population. 158,000. Elevation. 166 metres (545 feet) Open Location Code. 9G7WQCRR+56. OpenStreetMap ID. node 156167469. OpenStreetMap Feature. place=city. GeoNames ID. 563523.
Metallurgicheskii Zavod Electrostal AO (Russia) In 1993 "Elektrostal" was transformed into an open joint stock company. The factory occupies a leading position among the manufacturers of high quality steel. The plant is a producer of high-temperature nickel alloys in a wide variety. It has a unique set of metallurgical equipment: open induction ...